pthread实现梯形积分法
时间: 2023-07-18 14:04:54 浏览: 88
梯形积分法是一种数值积分方法,在计算机程序中可以使用pthread库来实现并行计算,提高计算效率。下面是一个使用pthread实现梯形积分法的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define MAX_THREADS 4
double a = 0, b = 1, h;
int n = 10000000;
double sum[MAX_THREADS];
int thread_count;
void* Trap(void* rank) {
long my_rank = (long) rank;
double local_a = a + my_rank * h * n / thread_count;
double local_b = local_a + h * n / thread_count;
double local_sum = (f(local_a) + f(local_b)) / 2.0;
int i;
for (i = 1; i < n / thread_count; i++) {
double x = local_a + i * h;
local_sum += f(x);
}
local_sum = local_sum * h;
sum[my_rank] = local_sum;
return NULL;
}
double f(double x) {
return x * x;
}
int main(int argc, char* argv[]) {
long thread;
pthread_t* thread_handles;
h = (b - a) / n;
thread_count = (int) strtol(argv[1], NULL, 10);
thread_handles = malloc(thread_count * sizeof(pthread_t));
for (thread = 0; thread < thread_count; thread++) {
pthread_create(&thread_handles[thread], NULL, Trap, (void*) thread);
}
for (thread = 0; thread < thread_count; thread++) {
pthread_join(thread_handles[thread], NULL);
}
double total_sum = 0;
int i;
for (i = 0; i < thread_count; i++) {
total_sum += sum[i];
}
printf("With n = %d trapezoids, our estimate\n", n);
printf("of the integral from %f to %f = %.15f\n", a, b, total_sum);
free(thread_handles);
return 0;
}
```
在上述代码中,我们使用了pthread_create()函数来创建线程,并使用pthread_join()函数等待线程执行完毕。在每个线程中,我们使用类似串行计算的方法来计算局部积分值,最后将所有线程的局部积分值相加得到最终结果。
需要注意的是,在使用pthread时需要注意线程安全,特别是对于全局变量的访问。本示例代码中,我们使用了sum数组来保存每个线程的局部积分值,避免了对全局变量的竞争访问。
阅读全文
相关推荐










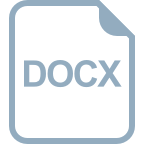