tensorflow对pascalvoc标注格式的数据进行训练
时间: 2023-09-09 19:14:34 浏览: 100
首先,需要将Pascal VOC标注格式的数据转换成TensorFlow支持的格式,可以使用一些开源工具来完成这个转换,例如`tfrecord-generator`。接着,可以使用TensorFlow的API来加载和训练数据集。
以下是一个简单的示例代码,用于加载和训练Pascal VOC数据集:
```python
import tensorflow as tf
# 加载tfrecord数据集
def load_dataset(tfrecord_path):
dataset = tf.data.TFRecordDataset(tfrecord_path)
# 解析tfrecord文件中的数据
feature_description = {
'image': tf.io.FixedLenFeature([], tf.string),
'label': tf.io.FixedLenFeature([], tf.string),
'width': tf.io.FixedLenFeature([], tf.int64),
'height': tf.io.FixedLenFeature([], tf.int64)
}
def _parse_function(example_proto):
parsed_features = tf.io.parse_single_example(example_proto, feature_description)
image = tf.io.decode_jpeg(parsed_features['image'], channels=3)
label = tf.io.decode_raw(parsed_features['label'], tf.uint8)
width = parsed_features['width']
height = parsed_features['height']
return (image, label), (width, height)
return dataset.map(_parse_function)
# 训练模型
def train_model(dataset):
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, (3, 3), activation='relu', input_shape=(None, None, 3)),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(10, activation='softmax')
])
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
model.fit(dataset, epochs=10)
# 加载数据集
train_dataset = load_dataset('/path/to/train.tfrecord')
test_dataset = load_dataset('/path/to/test.tfrecord')
# 训练模型
train_model(train_dataset)
```
需要注意的是,以上示例代码只是一个简单的例子,需要根据具体的任务和数据集进行修改和调整。
阅读全文
相关推荐
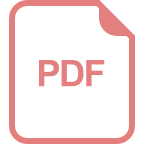
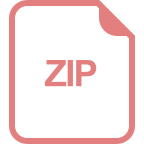

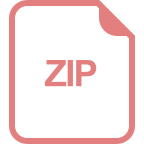
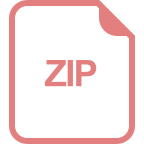
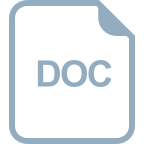
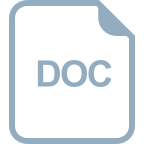
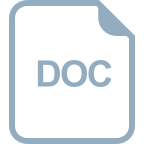
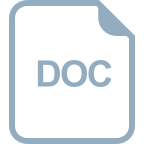
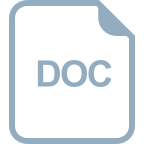
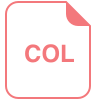
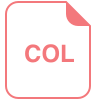
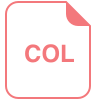
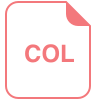
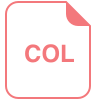




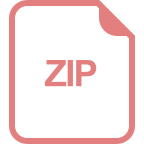