把这段代码从c++转换为c语言#include <iostream> #include <algorithm> #include <cmath> using namespace std; struct Person { string name; int height; }; bool cmp(Person p1, Person p2) { if (p1.height != p2.height) { return p1.height < p2.height; } else { return p1.name > p2.name; } } int main() { Person people[15]; int count = 0; while (true) { cin >> people[count].name; if (people[count].name == "#") { break; } cin >> people[count].height; count++; } sort(people, people + count, cmp); int mid = count / 2; if (count % 2 == 1) { cout << "mid height=" << people[mid].height << ",name=" << people[mid].name << endl; } else { cout << "mid height=" << (people[mid - 1].height + people[mid].height) / 2.0 << endl; cout << "first person:" << people[mid - 1].name << "," << people[mid - 1].height << endl; cout << "second person:" << people[mid].name << "," << people[mid].height << endl; } for (int i = 0; i < count; i++) { cout << people[i].name << " " << people[i].height << endl; } return 0; }
时间: 2024-03-05 19:53:59 浏览: 122
以下是将该C++代码转换为C语言代码的过程:
```c
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
struct Person {
char name[20];
int height;
};
int cmp(const void* p1, const void* p2) {
struct Person* person1 = (struct Person*) p1;
struct Person* person2 = (struct Person*) p2;
if (person1->height != person2->height) {
return person1->height - person2->height;
} else {
return strcmp(person2->name, person1->name);
}
}
int main() {
struct Person people[15];
int count = 0;
while (1) {
scanf("%s", people[count].name);
if (strcmp(people[count].name, "#") == 0) {
break;
}
scanf("%d", &people[count].height);
count++;
}
qsort(people, count, sizeof(struct Person), cmp);
int mid = count / 2;
if (count % 2 == 1) {
printf("mid height=%d,name=%s\n", people[mid].height, people[mid].name);
} else {
printf("mid height=%.1f\n", (people[mid - 1].height + people[mid].height) / 2.0);
printf("first person:%s,%d\n", people[mid - 1].name, people[mid - 1].height);
printf("second person:%s,%d\n", people[mid].name, people[mid].height);
}
for (int i = 0; i < count; i++) {
printf("%s %d\n", people[i].name, people[i].height);
}
return 0;
}
```
主要的变化包括:
1. 头文件改为 `<stdio.h>`, `<string.h>`, `<stdlib.h>`。
2. C语言中没有 `string` 类型,需要使用字符数组(char array)。
3. C语言中的函数 `scanf` 和 `printf` 替代了C++中的 `cin` 和 `cout`。
4. C语言中的比较函数 `qsort` 参数类型不同,需要进行相应的修改。
阅读全文
相关推荐
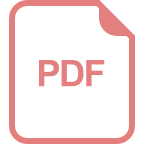
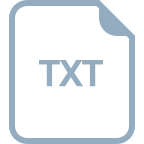







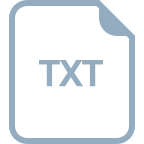
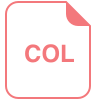
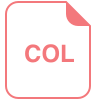





