const ctx = canvas.getContext('2d'); ctx.beginPath(); ctx.moveTo(0, 0); ctx.lineTo(50, 0); ctx.strokeStyle = '#ff0000'; ctx.lineWidth = 100; const ctx = canvas.getContext('2d'); ctx.beginPath(); ctx.moveTo(0, 0); ctx.lineTo(50, 0); ctx.strokeStyle = '#ff0000'; ctx.lineWidth = 100; ctx.stroke();
时间: 2024-04-20 14:25:56 浏览: 177
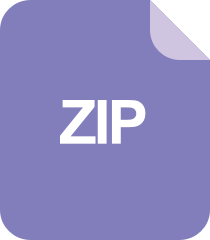
canvas-context:创建一个RenderingContext(2d,webgl,webgl2,bitmaprenderer,gpupresent),可以选择在屏幕外使用,以便在Worker中使用
这段代码使用Canvas API绘制了一条直线。首先,通过`canvas.getContext('2d')`方法获取了一个2D绘图上下文对象,将其赋值给`ctx`变量。
接下来,通过调用`ctx.beginPath()`方法开始一个新的路径。然后,使用`ctx.moveTo(0, 0)`将绘图起点移动到坐标(0, 0)。再使用`ctx.lineTo(50, 0)`从起点绘制一条直线到坐标(50, 0)。
接着,通过设置`ctx.strokeStyle`属性为`'#ff0000'`来指定线条的颜色为红色。使用`ctx.lineWidth`属性设置线条的宽度为100个单位。
最后,通过调用`ctx.stroke()`方法绘制出设置好的直线。
注意:代码中的第一行`const ctx = canvas.getContext('2d');`只需要出现一次即可,不需要重复出现。
阅读全文
相关推荐
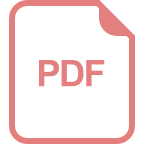



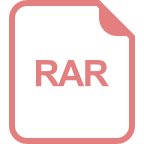
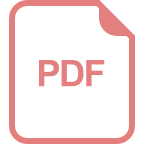
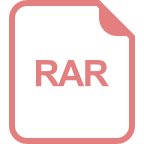
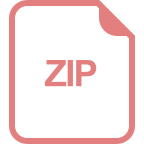
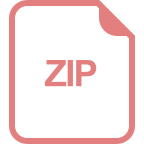
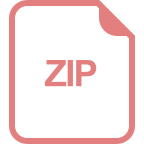
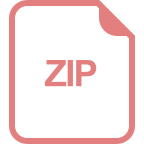
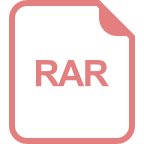
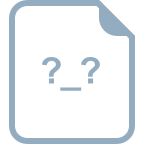
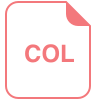
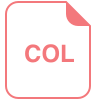
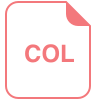
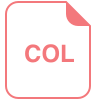