用np怎么实现
时间: 2023-08-17 20:10:34 浏览: 79
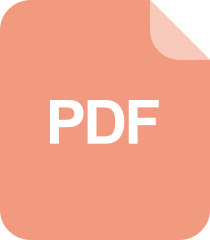
三旺NP301使用手册
如果你想用NumPy实现类ChatGPT的AI,你需要先构建一个文本数据集,然后使用NumPy将其转换为数字序列。然后,你需要使用NumPy定义一个神经网络模型,该模型可以接受数字序列作为输入,并生成相应的文本输出。
以下是一个简单的示例代码,说明了如何使用NumPy实现一个基本的文本生成器模型:
```python
import numpy as np
# 定义数据集
text = "This is a sample text for our NumPy based AI model."
chars = list(set(text))
char_to_idx = {ch:i for i,ch in enumerate(chars)}
idx_to_char = {i:ch for i,ch in enumerate(chars)}
text_len = len(text)
vocab_size = len(chars)
# 将文本转换为数字序列
text_as_int = np.array([char_to_idx[ch] for ch in text])
# 定义神经网络模型
hidden_size = 256
learning_rate = 0.1
Wxh = np.random.randn(hidden_size, vocab_size) * 0.01
Whh = np.random.randn(hidden_size, hidden_size) * 0.01
Why = np.random.randn(vocab_size, hidden_size) * 0.01
bh = np.zeros((hidden_size, 1))
by = np.zeros((vocab_size, 1))
# 定义softmax函数
def softmax(x):
e_x = np.exp(x - np.max(x))
return e_x / e_x.sum(axis=0)
# 定义前向传播函数
def forward_pass(inputs, hprev):
xs, hs, ys, ps = {}, {}, {}, {}
hs[-1] = np.copy(hprev)
for t in range(len(inputs)):
xs[t] = np.zeros((vocab_size,1))
xs[t][inputs[t]] = 1
hs[t] = np.tanh(np.dot(Wxh, xs[t]) + np.dot(Whh, hs[t-1]) + bh)
ys[t] = np.dot(Why, hs[t]) + by
ps[t] = softmax(ys[t])
return xs, hs, ys, ps, hs[len(inputs)-1]
# 定义损失函数
def loss_func(ps, targets):
loss = 0
for t in range(len(targets)):
loss += -np.log(ps[t][targets[t],0])
return loss
# 定义反向传播函数
def backward_pass(xs, hs, ys, ps, targets, hprev):
dWxh, dWhh, dWhy = np.zeros_like(Wxh), np.zeros_like(Whh), np.zeros_like(Why)
dbh, dby = np.zeros_like(bh), np.zeros_like(by)
dhnext = np.zeros_like(hprev)
for t in reversed(range(len(targets))):
dy = np.copy(ps[t])
dy[targets[t]] -= 1
dWhy += np.dot(dy, hs[t].T)
dby += dy
dh = np.dot(Why.T, dy) + dhnext
dhraw = (1 - hs[t]*hs[t]) * dh
dbh += dhraw
dWxh += np.dot(dhraw, xs[t].T)
dWhh += np.dot(dhraw, hs[t-1].T)
dhnext = np.dot(Whh.T, dhraw)
for dparam in [dWxh, dWhh, dWhy, dbh, dby]:
np.clip(dparam, -5, 5, out=dparam)
return dWxh, dWhh, dWhy, dbh, dby, hs[len(targets)-1]
# 定义训练函数
def train(text_as_int, char_to_idx, idx_to_char, vocab_size, hidden_size, learning_rate, num_epochs):
Wxh = np.random.randn(hidden_size, vocab_size) * 0.01
Whh = np.random.randn(hidden_size, hidden_size) * 0.01
Why = np.random.randn(vocab_size, hidden_size) * 0.01
bh = np.zeros((hidden_size, 1))
by = np.zeros((vocab_size, 1))
hprev = np.zeros((hidden_size, 1))
for epoch in range(num_epochs):
loss = 0
for i in range(0, text_len-1):
inputs = text_as_int[i:i+1]
targets = text_as_int[i+1:i+2]
xs, hs, ys, ps, hprev = forward_pass(inputs, hprev)
loss += loss_func(ps, targets)
dWxh, dWhh, dWhy, dbh, dby, hprev = backward_pass(xs, hs, ys, ps, targets, hprev)
Wxh -= learning_rate * dWxh
Whh -= learning_rate * dWhh
Why -= learning_rate * dWhy
bh -= learning_rate * dbh
by -= learning_rate * dby
if epoch % 10 == 0:
sample_ix = sample(hprev, inputs, 200)
txt = ''.join(idx_to_char[ix] for ix in sample_ix)
print('----\n %s \n----' % (txt, ))
print('Epoch: ', epoch, ' Loss: ', loss)
# 定义采样函数
def sample(h, seed_ix, n):
x = np.zeros((vocab_size, 1))
x[seed_ix] = 1
ixes = []
for t in range(n):
h = np.tanh(np.dot(Wxh, x) + np.dot(Whh, h) + bh)
y = np.dot(Why, h) + by
p = softmax(y)
ix = np.random.choice(range(vocab_size), p=p.ravel())
x = np.zeros((vocab_size, 1))
x[ix] = 1
ixes.append(ix)
return ixes
# 训练模型
train(text_as_int, char_to_idx, idx_to_char, vocab_size, hidden_size, learning_rate, num_epochs=100)
```
这是一个简单的模型,仅用于演示如何使用NumPy实现类ChatGPT的AI。如果你想要更复杂的模型,你需要深入学习NumPy和神经网络的相关知识。
阅读全文
相关推荐
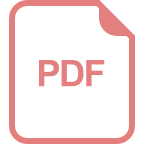
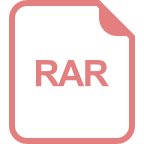
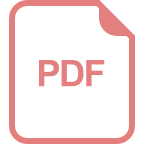
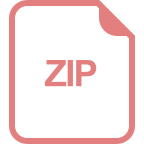
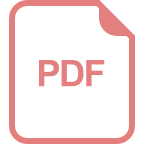
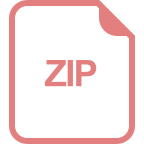










