STM32F103RC, 代码实现TFT屏驱动
时间: 2023-10-01 15:07:51 浏览: 74
以下是 STM32F103RC 实现 TFT 屏驱动的代码示例:
```c
#include "stm32f10x.h"
#include "tft.h"
void tft_write_command(uint8_t command) {
// 将命令写入TFT控制器
GPIO_ResetBits(GPIOA, GPIO_Pin_1); // 将RS引脚置为低电平表示写入命令
GPIO_ResetBits(GPIOA, GPIO_Pin_2); // 将WR引脚置为低电平表示开始写入
GPIO_Write(GPIOB, command); // 将命令写入数据总线
GPIO_SetBits(GPIOA, GPIO_Pin_2); // 将WR引脚置为高电平表示写入完成
}
void tft_write_data(uint8_t data) {
// 将数据写入TFT控制器
GPIO_SetBits(GPIOA, GPIO_Pin_1); // 将RS引脚置为高电平表示写入数据
GPIO_ResetBits(GPIOA, GPIO_Pin_2); // 将WR引脚置为低电平表示开始写入
GPIO_Write(GPIOB, data); // 将数据写入数据总线
GPIO_SetBits(GPIOA, GPIO_Pin_2); // 将WR引脚置为高电平表示写入完成
}
void tft_init(void) {
// 初始化TFT控制器
tft_write_command(0x01); // 软复位TFT控制器
tft_write_command(0x11); // 退出睡眠模式
tft_write_command(0x3A); // 设置像素格式为16位色深
tft_write_data(0x55);
tft_write_command(0x36); // 设置扫描方向为正常方向
tft_write_data(0xC0);
tft_write_command(0x29); // 打开显示
}
void tft_set_pixel(uint16_t x, uint16_t y, uint16_t color) {
// 设置指定像素的颜色
tft_write_command(0x2A); // 设置列地址
tft_write_data(x >> 8);
tft_write_data(x & 0xFF);
tft_write_data((x + 1) >> 8);
tft_write_data((x + 1) & 0xFF);
tft_write_command(0x2B); // 设置行地址
tft_write_data(y >> 8);
tft_write_data(y & 0xFF);
tft_write_data((y + 1) >> 8);
tft_write_data((y + 1) & 0xFF);
tft_write_command(0x2C); // 设置写入像素数据
tft_write_data(color >> 8);
tft_write_data(color & 0xFF);
}
```
需要注意的是,在使用该代码之前,需要先定义引脚和端口。例如,我们可以将TFT屏的RS引脚连接到PA1引脚,WR引脚连接到PA2引脚,数据总线连接到PB0-PB7引脚。因此,我们需要在代码中定义这些引脚和端口:
```c
// 定义引脚和端口
#define TFT_RS_Pin GPIO_Pin_1
#define TFT_RS_Port GPIOA
#define TFT_WR_Pin GPIO_Pin_2
#define TFT_WR_Port GPIOA
#define TFT_Data_Port GPIOB
// 初始化引脚和端口
void tft_gpio_init(void) {
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_GPIOB, ENABLE);
// 配置RS引脚
GPIO_InitStructure.GPIO_Pin = TFT_RS_Pin;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(TFT_RS_Port, &GPIO_InitStructure);
// 配置WR引脚
GPIO_InitStructure.GPIO_Pin = TFT_WR_Pin;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(TFT_WR_Port, &GPIO_InitStructure);
// 配置数据总线
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0 | GPIO_Pin_1 | GPIO_Pin_2 | GPIO_Pin_3 | GPIO_Pin_4 | GPIO_Pin_5 | GPIO_Pin_6 | GPIO_Pin_7;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(TFT_Data_Port, &GPIO_InitStructure);
}
```
在使用该代码之前,需要先调用 `tft_gpio_init()` 函数初始化引脚和端口。然后可以调用 `tft_init()` 函数初始化TFT控制器。最后,可以使用 `tft_set_pixel()` 函数设置像素的颜色。
阅读全文
相关推荐
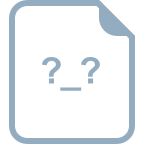

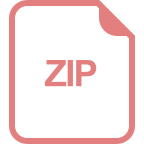
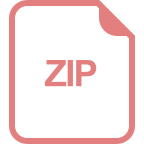
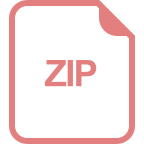
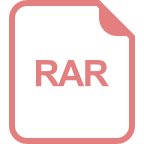
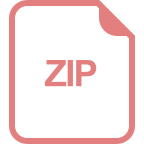
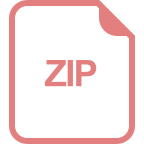
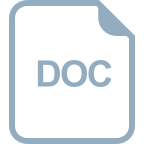
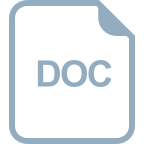
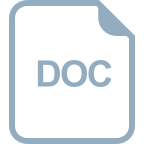
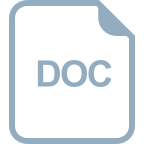
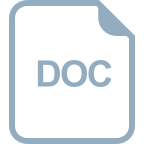
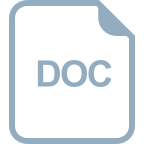
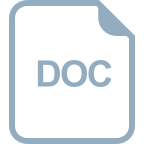
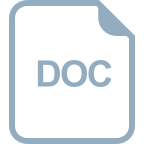
