分块查找但不用结构体的c语言代码
时间: 2023-10-14 20:04:26 浏览: 77
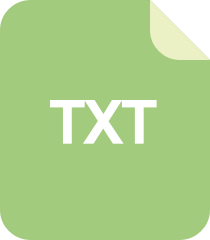
c语言实现分块查找
下面是一个用 C 语言实现的分块查找算法的示例代码,不使用结构体:
```c
#include <stdio.h>
// 定义分块大小为 3
#define BLOCK_SIZE 3
// 查找函数
int block_search(int *arr, int n, int key) {
int i, j;
// 计算块数
int block_num = n / BLOCK_SIZE + (n % BLOCK_SIZE ? 1 : 0);
// 在块中查找
for (i = 0; i < block_num; i++) {
// 如果查找的键值小于块的最后一个元素,则在当前块中查找
if (key <= arr[(i + 1) * BLOCK_SIZE - 1]) {
for (j = i * BLOCK_SIZE; j < (i + 1) * BLOCK_SIZE; j++) {
if (arr[j] == key) {
return j;
}
}
// 如果当前块中未找到,则直接返回
return -1;
}
}
// 如果在所有块中都未找到,则返回 -1
return -1;
}
int main() {
int arr[] = {1, 3, 5, 7, 9, 11, 13, 15, 17, 19};
int n = sizeof(arr) / sizeof(int);
int key = 11;
int index = block_search(arr, n, key);
if (index == -1) {
printf("未找到 %d\n", key);
} else {
printf("找到 %d, 下标为 %d\n", key, index);
}
return 0;
}
```
在这个示例代码中,我们定义了一个常量 `BLOCK_SIZE` 来表示每个块的大小。我们首先计算出整个数组中元素的个数 `n`,然后计算出块的数量 `block_num`,最后在块中查找给定键值 `key`。
如果查找的键值小于块的最后一个元素,则在当前块中查找,否则继续在下一个块中查找。如果在所有块中都未找到,则返回 -1。
希望这段代码能够帮助到你。
阅读全文
相关推荐
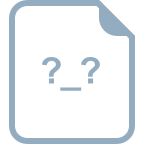
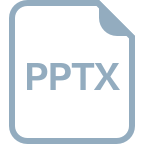
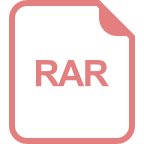
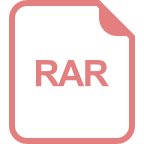
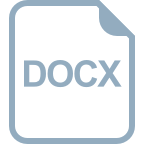
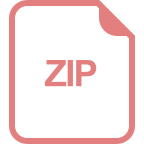
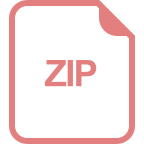
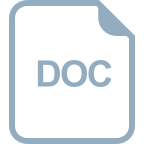
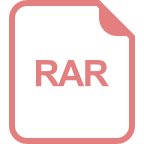
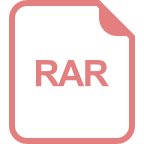
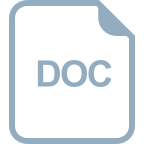
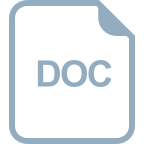
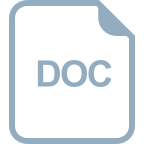
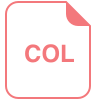
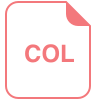

