C语言分块查找,但是有的块没有填满,应该怎么写代码
时间: 2024-09-17 18:05:34 浏览: 30
C语言中的分块查找,也称为动态数组或动态内存分配,如果遇到部分块未完全填满的情况,通常会涉及到链表或者动态内存管理。在这种场景下,你可以使用头指针(如`node`结构体,包含数据和指向下一个元素的指针)来表示每个未满的块。
以下是一个简单的示例,假设我们有一个动态分配的整数数组,并使用链表形式存储:
```c
typedef struct Node {
int data; // 数据项
struct Node* next; // 指向下一个节点的指针
} BlockNode;
BlockNode* head = NULL; // 总头指针
// 插入数据到特定块
void insert(int value, int block_size) {
BlockNode* newNode = (BlockNode*)malloc(sizeof(BlockNode));
if (newNode != NULL) {
newNode->data = value;
newNode->next = NULL;
// 找到当前块的位置
BlockNode* current = head;
for (int i = 0; i < block_size && current != NULL; ++i, current = current->next) {
if (current == NULL) { // 当前块结束,开始新块
newNode->next = head; // 新节点作为新块的起始
head = newNode;
break;
}
}
current->next = newNode; // 将新的节点插入适当位置
} else {
printf("Memory allocation failed.\n");
}
}
// 查找数据
int search(int value, int block_size) {
BlockNode* current = head;
while (current != NULL) {
if (current->data == value) {
return 1; // 数据找到,返回1
}
current = current->next;
// 如果到了一个空的块并且还没查完,说明未找到
if (current != NULL && current->next == NULL && current->data == 0) {
return -1; // 表示数据不在当前已分配的块中
}
}
return 0; // 数据没找到,返回0
}
阅读全文
相关推荐
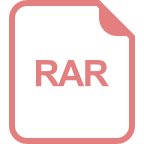















