怎么编写python代码实现:连接MongoDB数据库的两个表,输出字段
时间: 2024-03-21 21:43:36 浏览: 71
可以使用PyMongo库连接MongoDB数据库,并使用find()方法查询两个表并输出字段。
以下是一个基本的示例代码:
```python
import pymongo
# 连接MongoDB数据库
client = pymongo.MongoClient("mongodb://localhost:27017/")
# 选择数据库
db = client["mydatabase"]
# 选择两个表
collection1 = db["customers"]
collection2 = db["orders"]
# 查询两个表
result1 = collection1.find()
result2 = collection2.find()
# 输出字段
for x in result1:
print(x["name"])
for y in result2:
print(y["product"])
```
代码中,我们首先使用pymongo.MongoClient()方法连接MongoDB数据库,然后选择我们要使用的数据库。接着,我们选择了两个表:customers和orders,并使用find()方法查询它们。最后,我们使用for循环逐个输出查询结果中的字段。
相关问题
怎么编写python代码实现:把MongoDB数据库的两个表进行连接,查询连接后的字段信息
在MongoDB中,可以使用聚合(aggregate)操作连接两个或更多的集合。使用PyMongo库可以方便地在Python中执行聚合操作。
以下是一个基本的示例代码:
```python
import pymongo
# 连接MongoDB数据库
client = pymongo.MongoClient("mongodb://localhost:27017/")
# 选择数据库
db = client["mydatabase"]
# 选择两个表
collection1 = db["customers"]
collection2 = db["orders"]
# 连接两个表并查询连接后的字段信息
result = collection1.aggregate([
{
"$lookup":
{
"from": "orders",
"localField": "customer_id",
"foreignField": "customer_id",
"as": "orders"
}
}
])
# 输出连接后的字段信息
for x in result:
print(x)
```
代码中,我们使用$pipeline操作符指定了一系列聚合阶段,其中第一个阶段是$lookup操作符,用于连接两个表。在$lookup操作符中,我们指定了要连接的表名称、本地字段和外部字段。最后,我们使用for循环逐个输出连接后的字段信息。
注意,连接两个表可能会产生较大的结果集,因此最好使用$limit操作符限制结果集的大小。此外,还可以使用$match操作符过滤结果集中的数据。
Python代码可以实现:将MongoDB数据库的一张表和SqlServer的一张表进行两表左连接后查询吗?怎么编写代码?
是的,可以使用 Python 编写代码实现 MongoDB 和 SQL Server 之间的两表左连接查询。具体步骤如下:
1. 使用 PyMongo 连接 MongoDB 数据库,并查询需要的数据。
```python
import pymongo
mongo_client = pymongo.MongoClient("mongodb://localhost:27017/")
mongo_db = mongo_client["mydatabase"]
mongo_collection = mongo_db["collection1"]
mongo_data = mongo_collection.find()
```
2. 使用 pyodbc 或 pymssql 连接 SQL Server 数据库,并查询需要的数据。
```python
import pyodbc
sql_server_connection = pyodbc.connect('Driver={SQL Server};'
'Server=server_name;'
'Database=database_name;'
'Trusted_Connection=yes;')
cursor = sql_server_connection.cursor()
cursor.execute("SELECT column1, column2, column3 FROM table1")
sql_server_data = cursor.fetchall()
```
3. 将 MongoDB 数据和 SQL Server 数据合并,并进行左连接查询。
```python
# 使用 pandas 将数据转换为 DataFrame 格式
import pandas as pd
mongo_df = pd.DataFrame(mongo_data)
sql_server_df = pd.DataFrame(sql_server_data)
# 将两个 DataFrame 进行左连接
result_df = pd.merge(mongo_df, sql_server_df, how='left', left_on='mongo_key', right_on='sql_server_key')
# 输出查询结果
print(result_df)
```
在上面的代码中,需要将 MongoDB 中的数据和 SQL Server 中的数据转换为 DataFrame 格式,并使用 pandas 的 merge 函数将两个 DataFrame 进行左连接。左连接的条件是 `left_on='mongo_key'` 和 `right_on='sql_server_key'`,即连接 MongoDB 数据库中的某个字段和 SQL Server 数据库中的某个字段,这两个字段需具有相同的值。
最后,输出左连接查询的结果 `result_df` 即可。
阅读全文
相关推荐
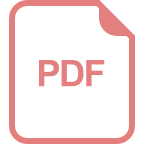
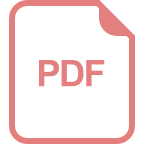
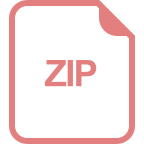
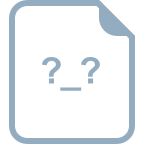
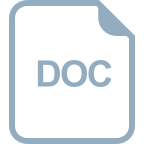
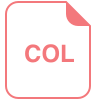
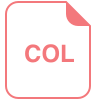
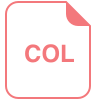
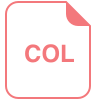
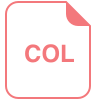
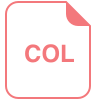
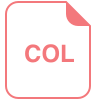
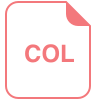
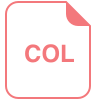
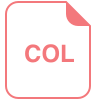
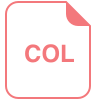
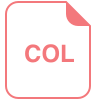