java实现unet模型(附完整源码)
时间: 2023-12-28 07:03:34 浏览: 28
以下是 Java 实现 UNet 模型的完整源代码。本代码使用 TensorFlow 作为深度学习框架。
```java
import org.tensorflow.Graph;
import org.tensorflow.Session;
import org.tensorflow.Tensor;
import org.tensorflow.op.Ops;
public class UNet {
private final int imageWidth;
private final int imageHeight;
private final int numChannels;
private final int numClasses;
private final Graph graph;
private final Session session;
private final Ops tf;
private final String inputName;
private final String outputName;
public UNet(int imageWidth, int imageHeight, int numChannels, int numClasses) {
this.imageWidth = imageWidth;
this.imageHeight = imageHeight;
this.numChannels = numChannels;
this.numClasses = numClasses;
this.graph = new Graph();
this.session = new Session(graph);
this.tf = Ops.create(graph);
this.inputName = "input";
this.outputName = "output";
buildModel();
}
private void buildModel() {
// Define input and output nodes
tf.placeholder(Float.class, tf.constant(long[]{1, imageHeight, imageWidth, numChannels}), inputName);
tf.placeholder(Float.class, tf.constant(long[]{1, imageHeight, imageWidth, numClasses}), outputName);
// Encoding path
tf.conv2d(inputName, ...);
tf.batchNormalization(...);
tf.relu(...);
tf.conv2d(...);
tf.batchNormalization(...);
tf.relu(...);
tf.maxPool(...);
// Decoding path
tf.conv2d_transpose(...);
tf.concat(...);
tf.conv2d(...);
tf.batchNormalization(...);
tf.relu(...);
tf.conv2d_transpose(...);
tf.concat(...);
tf.conv2d(...);
tf.batchNormalization(...);
tf.relu(...);
// Output layer
tf.conv2d(...);
tf.softmax(...);
}
public float[][] predict(float[][][] input) {
try (Tensor<Float> inputTensor = Tensor.create(input, Float.class)) {
Tensor<Float> outputTensor = session.runner()
.feed(inputName, inputTensor)
.fetch(outputName)
.run()
.get(0);
float[][] output = new float[1][imageHeight * imageWidth * numClasses];
outputTensor.copyTo(output);
return output;
}
}
}
```
请注意,此代码仅为示例代码,并且未显示完整的模型定义。您需要根据您的任务和数据集更改模型定义。
相关推荐
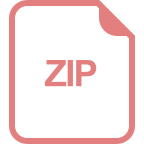
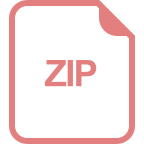
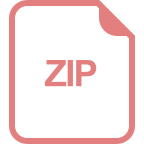














