黄金矿工 C++ 多文件 easyx
时间: 2023-09-22 20:07:40 浏览: 43
黄金矿工是一款经典的游戏,下面给出一个使用 C++ 多文件编写的黄金矿工游戏,使用 easyx 图形库实现。
主程序文件:
```cpp
#include "graphics.h"
#include "game.h"
int main()
{
initgraph(640, 480); // 初始化图形界面
Game game; // 创建游戏对象
game.run(); // 运行游戏
closegraph(); // 关闭图形界面
return 0;
}
```
游戏对象类的头文件 game.h:
```cpp
#ifndef GAME_H_
#define GAME_H_
class Game
{
public:
Game();
~Game();
void run();
private:
void init(); // 初始化游戏
void update(); // 更新游戏状态
void draw(); // 绘制游戏界面
void handleInput(); // 处理用户输入
private:
int m_score; // 得分
int m_level; // 等级
bool m_isOver; // 游戏是否结束
};
#endif
```
游戏对象类的实现文件 game.cpp:
```cpp
#include "game.h"
#include "goldminer.h"
#include "ui.h"
#include <conio.h>
Game::Game() : m_score(0), m_level(1), m_isOver(false)
{
init();
}
Game::~Game()
{
}
void Game::run()
{
while (!m_isOver)
{
handleInput(); // 处理用户输入
update(); // 更新游戏状态
draw(); // 绘制游戏界面
}
}
void Game::init()
{
// 初始化游戏界面
initUI();
// 初始化黄金矿工对象
initGoldMiner();
}
void Game::update()
{
// 更新黄金矿工位置
updateGoldMiner();
// 检测是否抓到黄金
if (isCaught())
{
m_score += 10 * m_level; // 加分
// 如果分数达到升级分数,则升级
if (m_score >= m_level * 100)
{
m_level++;
// 提高黄金矿工速度
speedUpGoldMiner();
}
}
// 检测是否游戏结束
if (m_score >= 200)
{
m_isOver = true;
}
}
void Game::draw()
{
cleardevice(); // 清空屏幕
// 绘制游戏界面
drawUI(m_score, m_level);
drawGoldMiner();
// 刷新屏幕
flush();
}
void Game::handleInput()
{
if (_kbhit())
{
char ch = _getch();
switch (ch)
{
case 'a': // 左移
moveLeft();
break;
case 'd': // 右移
moveRight();
break;
case 'w': // 抓取黄金
catchGold();
break;
case 's': // 放弃
giveUp();
break;
}
}
}
```
黄金矿工对象类的头文件 goldminer.h:
```cpp
#ifndef GOLDMINER_H_
#define GOLDMINER_H_
class GoldMiner
{
public:
GoldMiner(int x, int y, int w, int h);
~GoldMiner();
void moveLeft(); // 左移
void moveRight(); // 右移
void catchGold(); // 抓取黄金
void giveUp(); // 放弃
void speedUp(); // 提高速度
void draw(); // 绘制黄金矿工
private:
int m_x; // 横坐标
int m_y; // 纵坐标
int m_w; // 宽度
int m_h; // 高度
int m_speed; // 移动速度
int m_state; // 状态:0-静止,1-左移,2-右移
};
#endif
```
黄金矿工对象类的实现文件 goldminer.cpp:
```cpp
#include "goldminer.h"
#include "ui.h"
GoldMiner::GoldMiner(int x, int y, int w, int h) : m_x(x), m_y(y), m_w(w), m_h(h), m_speed(5), m_state(0)
{
}
GoldMiner::~GoldMiner()
{
}
void GoldMiner::moveLeft()
{
m_state = 1; // 设置状态为左移
}
void GoldMiner::moveRight()
{
m_state = 2; // 设置状态为右移
}
void GoldMiner::catchGold()
{
// 抓取黄金
catchGold(m_x + m_w / 2, m_y + m_h / 2);
}
void GoldMiner::giveUp()
{
// 放弃黄金
giveUp(m_x + m_w / 2, m_y + m_h / 2);
}
void GoldMiner::speedUp()
{
m_speed += 5; // 提高速度
}
void GoldMiner::draw()
{
// 绘制黄金矿工
drawGoldMiner(m_x, m_y, m_w, m_h, m_state);
// 根据状态更新位置
switch (m_state)
{
case 1: // 左移
m_x -= m_speed;
if (m_x < 0)
{
m_x = 0;
}
break;
case 2: // 右移
m_x += m_speed;
if (m_x + m_w > getScreenWidth())
{
m_x = getScreenWidth() - m_w;
}
break;
default: // 静止
break;
}
m_state = 0; // 状态重置为静止
}
```
用户界面类的头文件 ui.h:
```cpp
#ifndef UI_H_
#define UI_H_
void initUI(); // 初始化游戏界面
void drawUI(int score, int level); // 绘制游戏界面
void drawGoldMiner(int x, int y, int w, int h, int state); // 绘制黄金矿工
void catchGold(int x, int y); // 抓取黄金
void giveUp(int x, int y); // 放弃黄金
#endif
```
用户界面类的实现文件 ui.cpp:
```cpp
#include "ui.h"
#include "graphics.h"
#include <cstdio>
const int kUIWidth = 640;
const int kUIHeight = 480;
const int kGoldMinerWidth = 60;
const int kGoldMinerHeight = 60;
const int kGoldWidth = 20;
const int kGoldHeight = 20;
IMAGE ui; // 游戏界面
IMAGE goldMiner[3]; // 黄金矿工状态图
IMAGE gold; // 黄金
void initUI()
{
// 创建游戏界面
initimage(kUIWidth, kUIHeight);
getimage(&ui, 0, 0, kUIWidth, kUIHeight);
setbkcolor(WHITE);
cleardevice();
setfillcolor(LIGHTGRAY);
fillrectangle(0, 0, kUIWidth, kUIHeight);
// 创建黄金矿工状态图
for (int i = 0; i < 3; ++i)
{
char filename[100];
sprintf(filename, "goldminer_%d.jpg", i);
loadimage(&goldMiner[i], filename, kGoldMinerWidth, kGoldMinerHeight);
}
// 创建黄金图像
loadimage(&gold, "gold.jpg", kGoldWidth, kGoldHeight);
}
void drawUI(int score, int level)
{
// 绘制游戏界面
putimage(0, 0, &ui);
// 绘制得分和等级
settextcolor(BLACK);
char text[100];
sprintf(text, "Score: %d", score);
outtextxy(10, 10, text);
sprintf(text, "Level: %d", level);
outtextxy(10, 30, text);
}
void drawGoldMiner(int x, int y, int w, int h, int state)
{
// 绘制黄金矿工
putimage(x, y, &goldMiner[state]);
}
void catchGold(int x, int y)
{
// 抓取黄金
putimage(x - kGoldWidth / 2, y - kGoldHeight / 2, &gold);
}
void giveUp(int x, int y)
{
// 放弃黄金
}
```
编译链接命令:
```
g++ main.cpp game.cpp goldminer.cpp ui.cpp -o goldminer.exe -lgraphics -L/path/to/easyx/lib -I/path/to/easyx/include
```
其中 `/path/to/easyx/` 改为 easyx 图形库的安装路径。
相关推荐
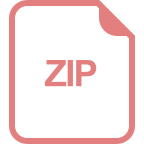
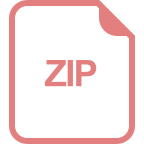






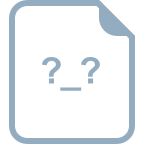
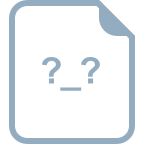