CShap 一个字符串是否为另一个字符串的子字符串 源代码
时间: 2023-12-25 17:04:02 浏览: 20
以下是C#代码,用于检查一个字符串是否为另一个字符串的子字符串:
```
string str1 = "Hello World";
string str2 = "World";
if (str1.Contains(str2))
{
Console.WriteLine("'{0}' 是 '{1}' 的子字符串", str2, str1);
}
else
{
Console.WriteLine("'{0}' 不是 '{1}' 的子字符串", str2, str1);
}
```
这段代码首先定义了两个字符串 `str1` 和 `str2`,然后使用 `Contains()` 方法检查 `str2` 是否为 `str1` 的子字符串。如果是,则输出相应的消息,否则输出另一条消息。
相关问题
CShap 一个字符串是否是另一个字符串的排列 源代码
以下是C#代码,用于检查一个字符串是否是另一个字符串的排列:
```csharp
using System;
public class Program
{
public static bool IsPermutation(string str1, string str2)
{
if (str1.Length != str2.Length)
return false;
int[] charCount = new int[256];
for (int i = 0; i < str1.Length; i++)
charCount[str1[i]]++;
for (int i = 0; i < str2.Length; i++)
{
charCount[str2[i]]--;
if (charCount[str2[i]] < 0)
return false;
}
return true;
}
public static void Main()
{
string str1 = "abcde";
string str2 = "edcba";
if (IsPermutation(str1, str2))
Console.WriteLine("'{0}' is a permutation of '{1}'", str1, str2);
else
Console.WriteLine("'{0}' is not a permutation of '{1}'", str1, str2);
}
}
```
该代码使用一个整数数组来计数每个字符在第一个字符串中出现的次数。然后,它遍历第二个字符串并递减相应字符的计数器。如果在任何时候计数器变为负数,则第二个字符串不是第一个字符串的排列,因此该函数将返回false。否则,如果在遍历第二个字符串后所有字符的计数器都为零,则第二个字符串是第一个字符串的排列,因此该函数将返回true。
CShap数字字符串排序 源代码
以下是一个用C#编写的数字字符串排序的源代码:
using System;
using System.Linq;
class Program
{
static void Main(string[] args)
{
string[] numbers = { "10", "9", "100", "200", "50" };
Console.WriteLine("排序前:");
foreach (string number in numbers)
{
Console.Write(number + " ");
}
Array.Sort(numbers, (a, b) => int.Parse(a).CompareTo(int.Parse(b)));
Console.WriteLine("\n排序后:");
foreach (string number in numbers)
{
Console.Write(number + " ");
}
Console.ReadLine();
}
}
该程序首先声明一个包含数字字符串的数组,然后使用Array.Sort方法进行排序。在这个例子中,使用lambda表达式作为比较器来确保以数字方式排序。最后,程序在控制台上打印排序前和排序后的数字字符串。
相关推荐
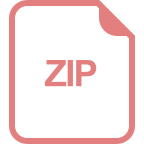












