c++LL(1)语法分析完整代码
时间: 2023-09-02 10:09:03 浏览: 95
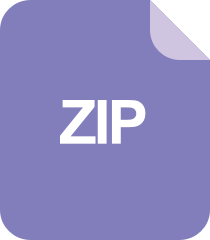
基于C++设计与实现的 LL1 语法分析程序(编译原理)【100012210】
下面是一个简单的 LL(1) 语法分析器的完整代码,可以解析一个简单的算术表达式:
```
#include <stdio.h>
#include <ctype.h>
char lookahead;
void match(char c) {
if (lookahead == c) {
lookahead = getchar();
} else {
fprintf(stderr, "syntax error\n");
exit(1);
}
}
int is_digit(char c) {
return isdigit(c);
}
int is_addop(char c) {
return c == '+' || c == '-';
}
int factor() {
int result;
if (lookahead == '(') {
match('(');
result = expression();
match(')');
} else if (is_digit(lookahead)) {
result = lookahead - '0';
match(lookahead);
} else {
fprintf(stderr, "syntax error\n");
exit(1);
}
return result;
}
int term() {
int result = factor();
while (lookahead == '*' || lookahead == '/') {
if (lookahead == '*') {
match('*');
result *= factor();
} else {
match('/');
result /= factor();
}
}
return result;
}
int expression() {
int result = term();
while (is_addop(lookahead)) {
if (lookahead == '+') {
match('+');
result += term();
} else {
match('-');
result -= term();
}
}
return result;
}
int main() {
lookahead = getchar();
int result = expression();
printf("%d\n", result);
return 0;
}
```
这个代码的核心是三个函数 `factor()`, `term()`, `expression()`,它们分别对应算术表达式的三个级别:“因子”、“项”和“表达式”。在实现这些函数时,我们使用了一个全局变量 `lookahead` 来保存当前分析的字符。`match()` 函数用来检查当前的字符是否与期望的字符匹配,如果匹配则读入下一个字符,否则输出语法错误信息并退出程序。`is_digit()` 和 `is_addop()` 函数用来判断当前字符是否为数字或加减号。
在 `factor()` 函数中,如果当前字符是左括号,则递归地调用 `expression()` 函数来处理括号内的表达式;如果当前字符是数字,则将其转换为整数并读入下一个字符;否则输出语法错误信息。在 `term()` 函数中,我们先处理 `factor()`,然后循环读入下一个字符,如果是乘号或除号,则执行相应的操作;否则跳出循环。在 `expression()` 函数中,我们先处理 `term()`,然后循环读入下一个字符,如果是加号或减号,则执行相应的操作;否则跳出循环。最后在 `main()` 函数中,我们读入第一个字符,并调用 `expression()` 函数来处理整个表达式,然后输出结果。
阅读全文
相关推荐
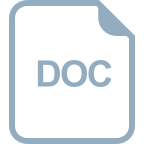
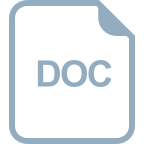
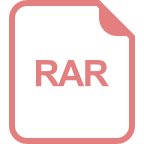
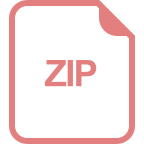
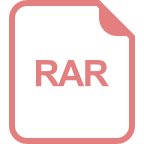
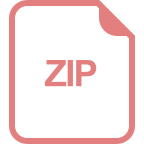
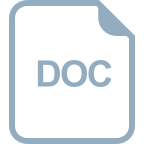
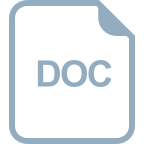







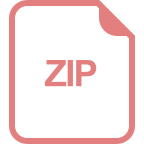
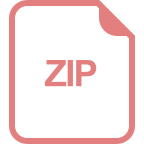