使用python开发一个象棋游戏
时间: 2024-02-03 19:03:41 浏览: 80
好的,下面是一个简单的Python象棋游戏的示例代码:
```python
class Chessboard:
def __init__(self):
self.board = [['r', 'n', 'b', 'q', 'k', 'b', 'n', 'r'],
['p', 'p', 'p', 'p', 'p', 'p', 'p', 'p'],
[' ', '.', ' ', '.', ' ', '.', ' ', '.'],
['.', ' ', '.', ' ', '.', ' ', '.', ' '],
[' ', '.', ' ', '.', ' ', '.', ' ', '.'],
['.', ' ', '.', ' ', '.', ' ', '.', ' '],
['P', 'P', 'P', 'P', 'P', 'P', 'P', 'P'],
['R', 'N', 'B', 'Q', 'K', 'B', 'N', 'R']]
self.turn = 'white'
def print_board(self):
for i in range(8):
print('\n')
for j in range(8):
print(self.board[i][j], end=' ')
print('\n')
def move(self, start, end):
x1, y1 = start
x2, y2 = end
piece = self.board[x1][y1]
if piece == ' ' or piece == '.':
print("There's no piece there!")
return False
if self.turn == 'white' and piece.islower():
print("It's not your turn!")
return False
if self.turn == 'black' and piece.isupper():
print("It's not your turn!")
return False
if piece == 'p':
if x1 - x2 == 1 and abs(y1 - y2) == 1 and self.board[x2][y2] != ' ' and self.board[x2][y2] != '.':
self.board[x2][y2] = 'p'
self.board[x1][y1] = ' '
elif x1 - x2 == 1 and y1 == y2 and self.board[x2][y2] == ' ':
self.board[x2][y2] = 'p'
self.board[x1][y1] = ' '
else:
print("Invalid move!")
return False
elif piece == 'P':
if x2 - x1 == 1 and abs(y1 - y2) == 1 and self.board[x2][y2] != ' ' and self.board[x2][y2] != '.':
self.board[x2][y2] = 'P'
self.board[x1][y1] = ' '
elif x2 - x1 == 1 and y1 == y2 and self.board[x2][y2] == ' ':
self.board[x2][y2] = 'P'
self.board[x1][y1] = ' '
else:
print("Invalid move!")
return False
else:
print("Invalid move!")
return False
self.turn = 'black' if self.turn == 'white' else 'white'
return True
chessboard = Chessboard()
chessboard.print_board()
while True:
start = input("Enter the starting position of the piece you want to move (e.g. 'a2'): ")
end = input("Enter the ending position of the piece you want to move (e.g. 'a4'): ")
x1, y1 = ord(start[0]) - 97, int(start[1]) - 1
x2, y2 = ord(end[0]) - 97, int(end[1]) - 1
if chessboard.move((x1, y1), (x2, y2)):
chessboard.print_board()
```
这个游戏使用了一个 `Chessboard` 类来表示棋盘,并且包含了一个 `move` 方法来移动棋子。在主循环中,程序会要求用户输入要移动的棋子的起始位置和终止位置,并且执行该移动。如果移动无效,程序会打印出错误信息并要求用户重新输入。
阅读全文
相关推荐
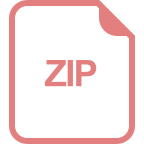
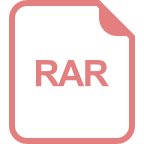
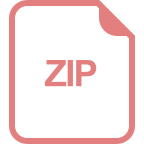















