hash join源码
时间: 2023-12-03 09:53:48 浏览: 168
Hash Join 是一种常见的关系型数据库中的 Join 算法,用于将两个表按照连接条件进行 Join 操作。下面是一个简单的 Hash Join 的源代码示例:
```c
/* 定义 hash 表结构体 */
typedef struct hash_entry {
int key;
int value;
struct hash_entry* next;
} hash_entry;
/* 定义 hash 表 */
typedef struct hash_table {
int size; /* hash 表长度 */
hash_entry** table; /* hash 表指针数组 */
} hash_table;
/* 创建 hash 表 */
hash_table* create_hash_table(int size) {
hash_table* table = (hash_table*) malloc(sizeof(hash_table));
table->size = size;
table->table = (hash_entry**) calloc(size, sizeof(hash_entry*));
return table;
}
/* 计算 hash 值 */
int hash(int key, int size) {
return key % size;
}
/* 向 hash 表中插入数据 */
void hash_insert(hash_table* table, int key, int value) {
int index = hash(key, table->size);
hash_entry* entry = table->table[index];
while (entry != NULL) {
if (entry->key == key) {
entry->value = value;
return;
}
entry = entry->next;
}
hash_entry* new_entry = (hash_entry*) malloc(sizeof(hash_entry));
new_entry->key = key;
new_entry->value = value;
new_entry->next = table->table[index];
table->table[index] = new_entry;
}
/* 从 hash 表中查找数据 */
int hash_lookup(hash_table* table, int key) {
int index = hash(key, table->size);
hash_entry* entry = table->table[index];
while (entry != NULL) {
if (entry->key == key) {
return entry->value;
}
entry = entry->next;
}
return -1;
}
/* Hash Join 算法 */
void hash_join(int* R, int* S, int n, int m) {
/* 创建 hash 表 */
hash_table* table = create_hash_table(n);
/* 构建 R 表的 hash 表 */
for (int i = 0; i < n; i++) {
hash_insert(table, R[i], i);
}
/* 查询 S 表中与 R 表相同的元素 */
for (int i = 0; i < m; i++) {
int index = hash_lookup(table, S[i]);
if (index != -1) {
printf("(%d, %d)\n", index, i);
}
}
}
```
上述代码中,我们定义了一个 hash 表结构体和 hash 表操作函数,然后使用 hash 表实现了一个简单的 Hash Join 算法。其中,我们通过创建 hash 表来存储 R 表的所有元素,然后遍历 S 表的所有元素,查找在 hash 表中是否存在相同元素,如果存在,就输出这两个元素的索引。
阅读全文
相关推荐
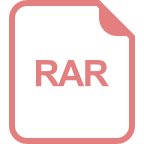
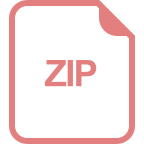
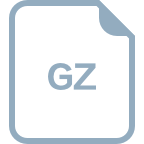








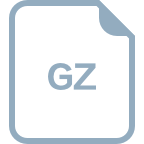
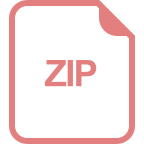
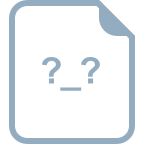
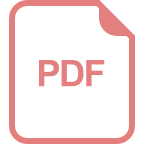
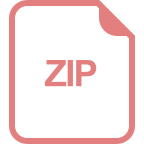
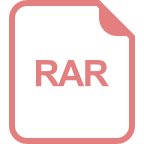
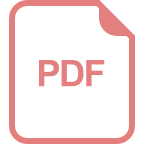
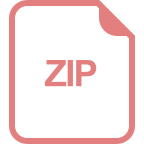