c++如何实现哈希表
时间: 2023-08-22 21:08:59 浏览: 119
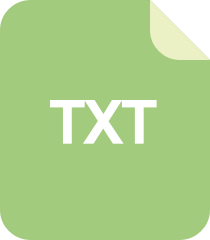
哈希表C++实现
C++中可以使用STL中的unordered_map来实现哈希表。unordered_map是一个基于哈希表实现的关联容器,可以快速地插入、查找和删除键值对。
下面是一个简单的示例代码,实现了一个存储字符串的哈希表:
```c++
#include <iostream>
#include <unordered_map>
#include <string>
using namespace std;
int main() {
// 创建哈希表
unordered_map<string, int> my_map;
// 插入键值对
my_map["apple"] = 1;
my_map["banana"] = 2;
my_map["orange"] = 3;
// 查找键值对
cout << "The value of 'apple' is " << my_map["apple"] << endl;
cout << "The value of 'banana' is " << my_map["banana"] << endl;
cout << "The value of 'orange' is " << my_map["orange"] << endl;
// 删除键值对
my_map.erase("orange");
// 遍历哈希表
for (auto it = my_map.begin(); it != my_map.end(); ++it) {
cout << it->first << " : " << it->second << endl;
}
return 0;
}
```
输出结果:
```
The value of 'apple' is 1
The value of 'banana' is 2
The value of 'orange' is 3
apple : 1
banana : 2
```
阅读全文
相关推荐
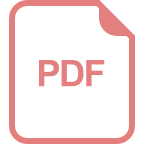
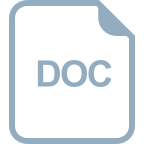
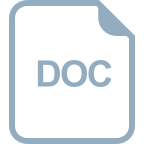
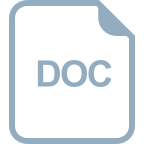
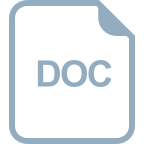
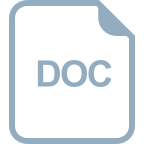
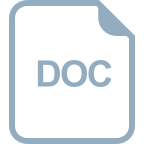
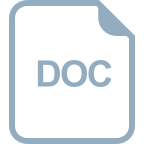
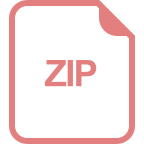

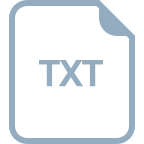
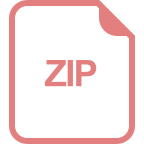
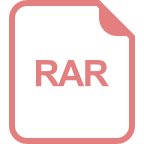
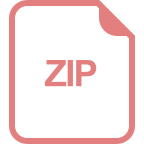
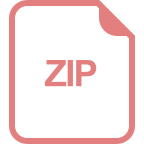
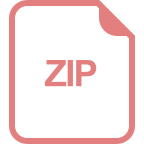