写出python一道题实现六个函数简单题目
时间: 2024-03-10 08:43:35 浏览: 25
好的,以下是一道题实现六个函数简单题的代码实现:
题目描述:
有一个类 `Rectangle`,它有两个属性 `width` 和 `height`,以及六个方法:
1. `set_width(width)`:将 `width` 属性的值设置为给定的值。
2. `set_height(height)`:将 `height` 属性的值设置为给定的值。
3. `get_area()`:返回此矩形的面积。
4. `get_perimeter()`:返回此矩形的周长。
5. `get_diagonal()`:返回此矩形的对角线长度。
6. `get_picture()`:返回一个字符串,表示用 `*` 组成的矩形图案。如果宽度或高度大于 50,则返回 `"Too big for picture."`。
请你用 Python 实现这个类 `Rectangle`。
```python
class Rectangle:
def __init__(self, width, height):
self.width = width
self.height = height
def set_width(self, width):
self.width = width
def set_height(self, height):
self.height = height
def get_area(self):
return self.width * self.height
def get_perimeter(self):
return 2 * (self.width + self.height)
def get_diagonal(self):
return (self.width ** 2 + self.height ** 2) ** 0.5
def get_picture(self):
if self.width > 50 or self.height > 50:
return "Too big for picture."
picture = ""
for _ in range(self.height):
picture += "*" * self.width + "\n"
return picture
def __str__(self):
return f"Rectangle(width={self.width}, height={self.height})"
```
以上代码实现了一个 `Rectangle` 类,包含了题目所要求的六个方法,可以通过实例化该类来使用这些方法。需要注意的是,`get_picture()` 方法返回的字符串中,每行都要以换行符 `\n` 结尾。同时,为了方便调试,我们还重写了该类的 `__str__()` 方法,使其返回一个字符串,表示该矩形的宽度和高度。
相关推荐
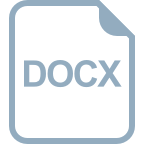
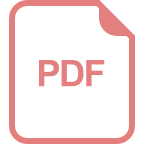
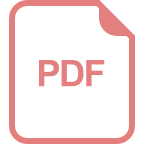














