用C++实现,将绝对路径转换成自定义QAbstractItemModel使用的index
时间: 2024-05-15 09:14:01 浏览: 229
可以使用QFileSystemModel类来实现绝对路径到QModelIndex的转换,具体操作如下:
```cpp
#include <QFileSystemModel>
#include <QFileInfo>
QString absolutePath = "/home/user/documents/example.txt";
// 创建QFileSystemModel对象
QFileSystemModel* model = new QFileSystemModel();
// 设置根目录
model->setRootPath("/");
// 获取文件信息
QFileInfo info(absolutePath);
// 获取文件路径
QString filePath = info.absoluteFilePath();
// 获取文件对应的QModelIndex
QModelIndex index = model->index(filePath);
```
使用上述代码,可以将绝对路径`/home/user/documents/example.txt`转换成QAbstractItemModel使用的QModelIndex对象。注意,这里使用的是QFileSystemModel类,如果你使用自定义的QAbstractItemModel类,需要根据自己的实现进行相应的更改。
相关问题
用C++实现,将绝对路径转换成QAbstractItemModel使用的index
QAbstractItemModel是Qt框架中的一个抽象类,用于实现数据模型。它提供了一些方法用于访问和修改数据,其中就包括index()方法,用于获取给定行列的QModelIndex对象。
下面是将绝对路径转换成QAbstractItemModel使用的index的示例代码:
```c++
#include <QAbstractItemModel>
#include <QDir>
QModelIndex pathToIndex(const QString& path, QAbstractItemModel* model)
{
QModelIndex index = model->index(0, 0); // 获取根节点的索引
QStringList parts = QDir::toNativeSeparators(path).split(QDir::separator()); // 将路径分割成部分
foreach (const QString& part, parts) {
int row = index.row();
int rowCount = model->rowCount(index); // 获取当前节点的子节点数
for (int i = 0; i < rowCount; ++i) {
QModelIndex childIndex = model->index(i, 0, index); // 获取当前节点的第i个子节点的索引
if (childIndex.data().toString() == part) { // 如果子节点的名称与路径中的部分相同
index = childIndex; // 更新当前节点的索引为该子节点的索引
break;
}
}
if (index.row() == row) { // 如果没有找到与路径中的部分相同的子节点
return QModelIndex(); // 返回无效索引
}
}
return index;
}
```
这里假设数据模型中每个节点只有一个名称,可以通过调用索引的data()方法获取。如果节点有多个属性,需要修改代码以匹配路径中的每一部分。
将绝对路径转换成QAbstractItemModel使用的index的C++完整代码
以下是将绝对路径转换为QAbstractItemModel使用的index的C++完整代码:
```c++
QModelIndex indexFromPath(const QString& path, QAbstractItemModel* model) {
QStringList nodes = path.split('/', QString::SkipEmptyParts);
QModelIndex index = QModelIndex();
for (int i = 0; i < nodes.size(); ++i) {
bool found = false;
for (int j = 0; j < model->rowCount(index); ++j) {
QModelIndex child = model->index(j, 0, index);
if (child.data().toString() == nodes[i]) {
index = child;
found = true;
break;
}
}
if (!found) {
return QModelIndex();
}
}
return index;
}
```
此函数接受一个QString形式的绝对路径和一个QAbstractItemModel指针。它将路径分割成节点,并在模型中搜索每个节点。如果找到节点,则将索引更新为该节点的索引。如果找不到节点,则返回无效索引。
阅读全文
相关推荐
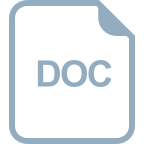
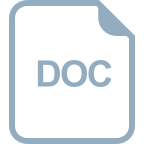
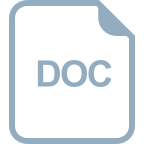













