怎样用c++ 部署pytorch模型
时间: 2023-08-03 11:04:02 浏览: 207
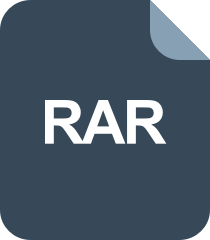
【pytorch】将yolov5模型通过openVINO2022部署至生产环境(二):C++版本博文对应源码
部署 PyTorch 模型需要使用 C++ API。以下是一些基本步骤:
1. 安装 PyTorch C++ 库。可以从官方网站下载预编译的包,也可以从源代码编译。
2. 加载 PyTorch 模型。使用 torch::jit::load() 函数加载训练好的模型。
3. 准备输入数据。根据模型的输入要求,准备输入张量。
4. 运行模型。使用 torch::jit::Module::forward() 函数运行模型。
5. 处理输出结果。根据模型的输出要求,处理输出张量。
以下是一个简单的示例代码,展示了如何加载并运行 PyTorch 模型:
```c++
#include <torch/script.h> // 包含 PyTorch C++ 库的头文件
#include <iostream>
int main() {
// 加载 PyTorch 模型
torch::jit::script::Module module;
try {
module = torch::jit::load("model.pt");
}
catch (const c10::Error& e) {
std::cerr << "Error loading the model\n";
return -1;
}
// 准备输入数据
torch::Tensor input = torch::ones({1, 3, 224, 224});
// 运行模型
auto output = module.forward({input}).toTensor();
// 处理输出结果
std::cout << output.slice(/*dim=*/1, /*start=*/0, /*end=*/5) << '\n';
return 0;
}
```
这个示例代码加载了名为 `model.pt` 的 PyTorch 模型,然后使用输入张量 `{1, 3, 224, 224}` 运行模型,并输出前 5 个元素。请注意,这只是一个简单的示例,实际上需要根据模型的具体情况来准备输入数据和处理输出结果。
阅读全文
相关推荐
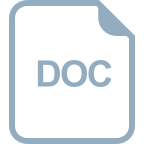
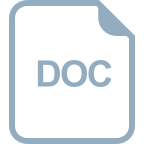



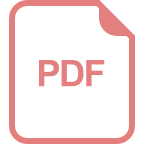



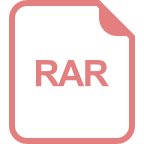
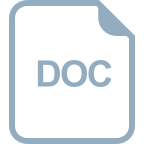
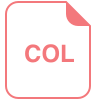





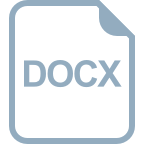