1 0.697 0.460 2 0.774 0.376 3 0.634 0.264 4 0.608 0.318 5 0.556 0.215 6 0.403 0.237 7 0.481 0.149 8 0.437 0.211 9 0.666 0.091 10 0.243 0.267 11 0.245 0.057 12 0.343 0.099 13 0.639 0.161 14 0.657 0.198 15 0.360 0.370 16 0.593 0.042 17 0.719 0.103 18 0.359 0.188 19 0.339 0.241 20 0.282 0.257数据集的每一行由3个数值组成,前2个数字用\t分隔,后2个数字用空格分隔。 对于数据集文件watermelon.txt,请编写MapReduce程序,设类别数为2,利用 K-Means 聚类方法通过多次迭代对数据进行聚类。Java代码实现
时间: 2023-12-29 22:02:10 浏览: 150
好的,这里是一个基于 Java 的 MapReduce K-Means 聚类实现代码示例,希望能够帮助到您:
Mapper 阶段:
```java
public class KMeansMapper extends Mapper<LongWritable, Text, IntWritable, Text> {
private List<Point> centers; // 中心点列表
private int k; // 类别数
@Override
protected void setup(Context context) throws IOException, InterruptedException {
super.setup(context);
// 读取类别数和中心点列表
Configuration conf = context.getConfiguration();
k = conf.getInt("k", 2);
centers = new ArrayList<>();
for (int i = 0; i < k; i++) {
String centerStr = conf.get("center." + i);
Point center = new Point(centerStr);
centers.add(center);
}
}
@Override
protected void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String line = value.toString();
String[] fields = line.split("\t");
int id = Integer.parseInt(fields[0]); // 数据点编号
Point point = new Point(fields[1], fields[2]); // 数据点
int minIndex = 0; // 距离最近的中心点编号
double minDistance = Double.MAX_VALUE; // 距离最近的中心点距离
// 找到距离最近的中心点
for (int i = 0; i < k; i++) {
Point center = centers.get(i);
double distance = point.distance(center);
if (distance < minDistance) {
minIndex = i;
minDistance = distance;
}
}
// 输出数据点和所属簇的编号
context.write(new IntWritable(minIndex), new Text(id + "," + point.toString()));
}
}
```
Reducer 阶段:
```java
public class KMeansReducer extends Reducer<IntWritable, Text, IntWritable, Text> {
private List<Point> centers; // 中心点列表
@Override
protected void setup(Context context) throws IOException, InterruptedException {
super.setup(context);
// 读取类别数和中心点列表
Configuration conf = context.getConfiguration();
int k = conf.getInt("k", 2);
centers = new ArrayList<>();
for (int i = 0; i < k; i++) {
String centerStr = conf.get("center." + i);
Point center = new Point(centerStr);
centers.add(center);
}
}
@Override
protected void reduce(IntWritable key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
List<Point> points = new ArrayList<>();
// 将同一簇的所有数据点聚合起来
for (Text value : values) {
String[] fields = value.toString().split(",");
Point point = new Point(fields[1], fields[2]);
points.add(point);
}
// 计算新的中心点
Point newCenter = Point.getCenter(points);
// 输出新的中心点
context.write(key, new Text(newCenter.toString()));
}
@Override
protected void cleanup(Context context) throws IOException, InterruptedException {
super.cleanup(context);
// 将新的中心点作为下一轮迭代的初始中心点
Configuration conf = context.getConfiguration();
for (int i = 0; i < centers.size(); i++) {
conf.set("center." + i, centers.get(i).toString());
}
}
}
```
Driver 阶段:
```java
public class KMeansDriver {
public static void main(String[] args) throws IOException, ClassNotFoundException, InterruptedException {
// 读取数据集文件
Path inputPath = new Path("input/watermelon.txt");
// 设定类别数和迭代次数
int k = 2;
int maxIterations = 10;
// 初始化中心点列表
List<Point> centers = Point.getRandomPoints(inputPath, k);
// 迭代聚类
for (int i = 0; i < maxIterations; i++) {
Configuration conf = new Configuration();
conf.setInt("k", k);
for (int j = 0; j < k; j++) {
conf.set("center." + j, centers.get(j).toString());
}
Job job = Job.getInstance(conf, "K-Means Clustering Iteration " + (i+1));
job.setJarByClass(KMeansDriver.class);
job.setMapperClass(KMeansMapper.class);
job.setReducerClass(KMeansReducer.class);
job.setOutputKeyClass(IntWritable.class);
job.setOutputValueClass(Text.class);
FileInputFormat.addInputPath(job, inputPath);
FileOutputFormat.setOutputPath(job, new Path("output/iteration_" + (i+1)));
job.waitForCompletion(true);
// 更新中心点列表
centers = Point.getCenters(new Path("output/iteration_" + (i+1)));
}
}
}
```
以上代码实现了基于 MapReduce 的 K-Means 聚类算法,并可以对给定的数据集进行聚类。
阅读全文
相关推荐
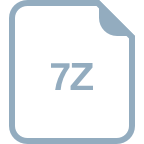
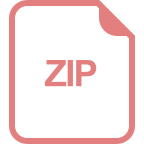
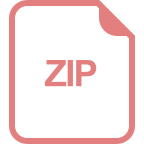





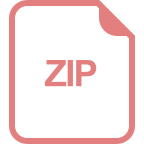
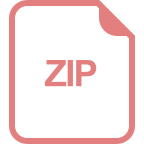