springboot配置扫描resource下的配置的xml文件
时间: 2024-03-26 08:40:51 浏览: 76
要让Spring Boot扫描resource下的配置的xml文件,你可以使用@ImportResource注解。在你的Spring Boot应用主类上添加这个注解,并指定你的xml文件的路径,例如:
```java
@SpringBootApplication
@ImportResource("classpath:applicationContext.xml")
public class YourApplication {
public static void main(String[] args) {
SpringApplication.run(YourApplication.class, args);
}
}
```
这将会让Spring Boot扫描classpath下的applicationContext.xml文件,并将其中定义的bean加载到Spring容器中。你可以根据自己的情况修改注解中的路径。
相关问题
SpringBoot 加载 hbm.xml
在 Spring Boot 中,我们可以通过配置 `LocalSessionFactoryBean` Bean 来加载 Hibernate 的配置文件和映射文件。下面是一个示例配置:
```java
@Configuration
@EnableTransactionManagement
public class HibernateConfig {
@Bean
public LocalSessionFactoryBean sessionFactory() {
LocalSessionFactoryBean sessionFactory = new LocalSessionFactoryBean();
sessionFactory.setDataSource(dataSource());
sessionFactory.setPackagesToScan("com.example.demo.entity");
sessionFactory.setHibernateProperties(hibernateProperties());
sessionFactory.setMappingLocations(new ClassPathResource("com/example/demo/entity/User.hbm.xml"));
return sessionFactory;
}
@Bean
public DataSource dataSource() {
DriverManagerDataSource dataSource = new DriverManagerDataSource();
dataSource.setDriverClassName("com.mysql.jdbc.Driver");
dataSource.setUrl("jdbc:mysql://localhost:3306/demo?useUnicode=true&characterEncoding=utf-8&serverTimezone=GMT%2B8");
dataSource.setUsername("root");
dataSource.setPassword("password");
return dataSource;
}
private final Properties hibernateProperties() {
Properties hibernateProperties = new Properties();
hibernateProperties.setProperty("hibernate.hbm2ddl.auto", "update");
hibernateProperties.setProperty("hibernate.dialect", "org.hibernate.dialect.MySQL5Dialect");
hibernateProperties.setProperty("hibernate.show_sql", "true");
return hibernateProperties;
}
}
```
在上面的配置中,我们通过 `setMappingLocations` 方法设置了 Hibernate 的映射文件位置。这里我们将 `User.hbm.xml` 文件放在了 `com/example/demo/entity/` 目录下,所以需要设置为 `new ClassPathResource("com/example/demo/entity/User.hbm.xml")`。如果有多个映射文件,可以设置多个 `ClassPathResource` 对象。当然,你也可以使用 `PathMatchingResourcePatternResolver` 来扫描指定目录下的所有映射文件。最后,将 `LocalSessionFactoryBean` Bean 注入到其他需要使用 Hibernate 的 Bean 中即可。
springboot自动化配置原理
Spring Boot 的自动化配置原理主要是基于依赖注入(Dependency Injection, DI)和配置文件的自动扫描。其核心思想是尽可能减少手动配置,让用户专注于业务逻辑。
1. **依赖注入**:Spring Boot 使用 BeanFactory 或者 ApplicationContext 来管理应用的组件,如控制器、服务、Repository 等。当需要使用某个服务时,而不是硬编码创建,而是通过注解如 `@Autowired` 或 `@Resource` 注入,Spring会自动查找并创建对应的bean实例。
2. **自动配置**:Spring Boot 提供了一系列的自动配置类(AutoConfiguration classes),它们会在运行时检查是否存在特定的条件(如某些依赖库的存在),然后自动配置相应的功能模块。例如,如果你的应用启用了 Web 服务,Spring Boot 就会自动配置 Servlet 和 WebMvc。
3. **YAML 或 XML 配置**:虽然默认情况下Spring Boot倾向于简化配置,但仍支持 YAML 或 XML 配置文件,用户可以根据需求自定义一些配置项。通过 @ConfigurationProperties 注解可以将配置映射到 Java 对象属性上。
4. **基于注解的配置**:许多常见的配置都可以通过注解完成,比如 @EnableAutoConfiguration 可以开启自动配置,@ComponentScan 则指定 Spring 扫描哪些包下的 beans。
阅读全文
相关推荐
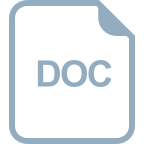
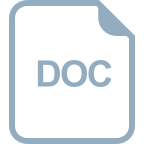
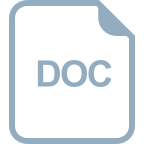
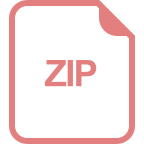
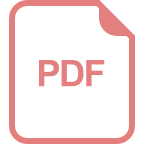
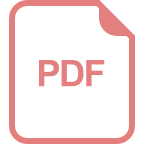
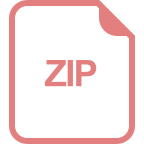
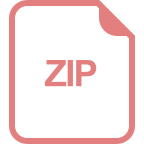
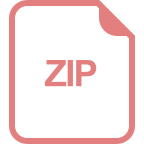
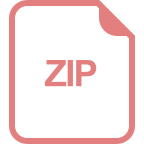
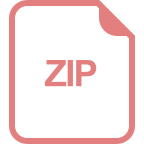





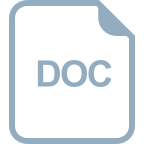