python中脑电选取一个marker进行2s分段代码
时间: 2023-07-23 18:08:30 浏览: 106
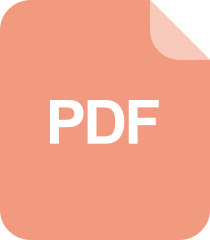
python通过文本在一个图中画多条线的实例
假设你的脑电数据存储在一个名为`eeg_data`的numpy数组中,采样频率为`fs`,你可以按照以下步骤选取一个marker进行2秒分段:
1. 找到marker出现的位置。假设你的marker值为`1`,可以使用`np.where()`函数找到所有`1`出现的位置:
```python
marker_indices = np.where(eeg_data == 1)[0]
```
2. 选择一个marker位置作为起点。假设你想选择第一个marker位置作为起点,可以使用`marker_indices[0]`。
3. 计算起点和终点的索引。假设你想选择2秒的时间窗口,那么终点索引可以计算为起点索引加上2秒对应的样本数:
```python
window_size = 2 # seconds
window_size_samples = int(window_size * fs)
start_index = marker_indices[0]
end_index = start_index + window_size_samples
```
4. 检查是否超出了数据范围。如果终点索引超出了数据范围,需要调整终点索引。可以使用`min()`函数确保终点索引不会超出数据范围:
```python
end_index = min(end_index, len(eeg_data))
```
5. 提取数据。使用起点索引和终点索引从数据中提取出相应的时间窗口:
```python
eeg_window = eeg_data[start_index:end_index]
```
完整的代码如下:
```python
import numpy as np
# assume eeg_data is a numpy array containing EEG data
# assume fs is the sampling frequency of the EEG data
eeg_data = ...
fs = ...
# find marker indices
marker_indices = np.where(eeg_data == 1)[0]
# choose first marker index as starting point
start_index = marker_indices[0]
# calculate window size in samples
window_size = 2 # seconds
window_size_samples = int(window_size * fs)
# calculate end index
end_index = start_index + window_size_samples
end_index = min(end_index, len(eeg_data))
# extract window
eeg_window = eeg_data[start_index:end_index]
```
注意,这里假设marker值为`1`,如果你的marker值不同,需要相应地修改代码。
阅读全文
相关推荐
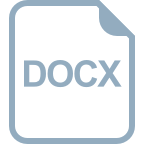
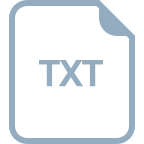

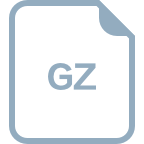
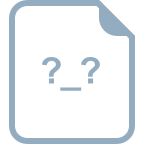
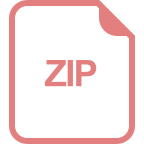
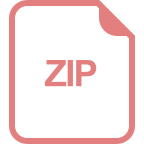










