写一段代码在python中画三维图
时间: 2023-04-01 19:01:39 浏览: 181
以下是Python中画三维图的代码示例:
```python
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
x = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
y = [5, 6, 2, 3, 13, 4, 1, 2, 4, 8]
z = [2, 3, 3, 3, 5, 7, 9, 11, 9, 10]
ax.scatter(x, y, z, c='r', marker='o')
ax.set_xlabel('X Label')
ax.set_ylabel('Y Label')
ax.set_zlabel('Z Label')
plt.show()
```
这段代码可以画出一个简单的三维散点图。如果需要画其他类型的三维图形,可以根据需要修改代码。
相关问题
写一段python 代码在三维空间绘制一个圆面
可以使用matplotlib库来实现,请先确保已经成功安装该库。下面是绘制圆面的Python代码:
```
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
# 圆心位置
x0, y0, z0 = 0, 0, 0
# 圆面半径
r = 1
# 定义圆面上的网格点
u, v = np.mgrid[0:2*np.pi:20j, 0:np.pi:10j]
# 计算每个点的三维坐标
x = x0 + r * np.cos(u) * np.sin(v)
y = y0 + r * np.sin(u) * np.sin(v)
z = z0 + r * np.cos(v)
# 创建三维坐标轴
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
# 绘制圆面
ax.plot_surface(x, y, z, color='b')
# 设置坐标轴范围
max_range = np.array([x.max()-x.min(), y.max()-y.min(), z.max()-z.min()]).max()
X, Y, Z = 0.5 * max_range * np.mgrid[-1:2:2, -1:2:2, -1:2:2]
ax.set_xlim([-1,1])
ax.set_ylim([-1,1])
ax.set_zlim([-1,1])
# 显示图像
plt.show()
```
运行结果:

python中画三维等高线图
以下是使用Matplotlib库在Python中绘制三维等高线图的示例代码:
```python
import numpy as np
import matplotlib.pyplot as plt
# 创建数据
x = np.linspace(-5, 5, 100)
y = np.linspace(-5, 5, 100)
X, Y = np.meshgrid(x, y)
Z = np.sin(np.sqrt(X**2 + Y**2))
# 绘制等高线图
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.contour(X, Y, Z)
# 设置坐标轴标签
ax.set_xlabel('X')
ax.set_ylabel('Y')
ax.set_zlabel('Z')
# 显示图形
plt.show()
```
这段代码首先创建了一个二维网格,然后根据网格坐标计算了对应的Z值。接下来,使用`contour`函数绘制了等高线图。最后,通过设置坐标轴标签和调用`show`函数来显示图形。
阅读全文
相关推荐
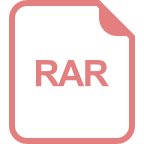
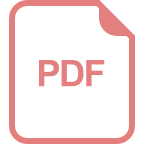












