aes128python实现
时间: 2023-07-28 13:06:38 浏览: 85
以下是使用Python实现AES-128加密和解密的示例代码:
```python
from Crypto.Cipher import AES
import base64
# 密钥(key)长度必须是16(AES-128)、24(AES-192)或32(AES-256)Bytes长度
key = '1234567890123456'.encode('utf-8')
iv = '1234567890123456'.encode('utf-8')
# 加密函数,如果text不足16位就用空格补足为16位,如果大于16但是不是16的倍数,就补足为16的倍数。
def encrypt(text):
cryptor = AES.new(key, AES.MODE_CBC, iv)
length = 16
count = len(text.encode('utf-8'))
if(count % length != 0):
add = length - (count % length)
else:
add = 0
text = text + ('\0' * add)
ciphertext = cryptor.encrypt(text.encode('utf-8'))
return base64.b64encode(ciphertext).decode('utf-8')
# 解密后,去掉补足的空格用strip() 去掉
def decrypt(text):
cryptor = AES.new(key, AES.MODE_CBC, iv)
plain_text = cryptor.decrypt(base64.b64decode(text)).decode('utf-8')
return plain_text.rstrip('\0')
if __name__ == '__main__':
# 测试加解密
e = encrypt("hello world")
print(e)
d = decrypt(e)
print(d)
```
需要注意的是,如果`text`不是16的倍数,就需要进行补齐,这里使用空格进行补齐,解密后再去掉补齐的空格。同时,加密后的密文需要进行base64编码,以便于传输和保存。
阅读全文
相关推荐
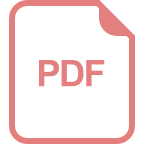









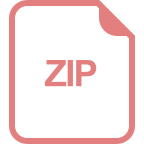
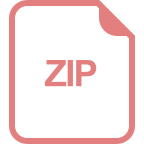
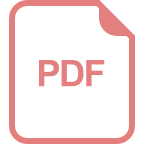