编程实现:从键盘输入任意一个字符串,统计大写英文字母和小写英文字母的个数。
时间: 2024-05-14 10:14:06 浏览: 81
```
#include <stdio.h>
#include <ctype.h>
int main() {
char str[100];
int upper = 0, lower = 0, i = 0;
printf("请输入一个字符串:");
fgets(str, 100, stdin);
while (str[i] != '\0') {
if (isupper(str[i])) {
upper++;
} else if (islower(str[i])) {
lower++;
}
i++;
}
printf("大写字母个数:%d\n", upper);
printf("小写字母个数:%d\n", lower);
return 0;
}
```
相关问题
编程实现:从键盘输入任意一个字符串,统计大写英文字母和小写英文字母的个数
```python
string = input("请输入一个字符串:")
count_upper = 0 # 大写字母个数
count_lower = 0 # 小写字母个数
for char in string:
if char.isupper(): # 判断是否为大写字母
count_upper += 1
elif char.islower(): # 判断是否为小写字母
count_lower += 1
print("大写字母个数:", count_upper)
print("小写字母个数:", count_lower)
```
样例输入:
```
Hello World!
```
样例输出:
```
大写字母个数: 2
小写字母个数: 8
```
键盘输入任意一个字符串,分别输出其中所含大写英文字母、小写英文字母、数字、和其它字符的个数;
好的,我已经明白你的问题了。我可以为你提供一个Python代码来解决这个问题:
```
string = input("请输入一个字符串:")
uppercase_count = 0
lowercase_count = 0
digit_count = 0
other_count = 0
for char in string:
if char.isupper():
uppercase_count += 1
elif char.islower():
lowercase_count += 1
elif char.isdigit():
digit_count += 1
else:
other_count += 1
print("大写字母个数:", uppercase_count)
print("小写字母个数:", lowercase_count)
print("数字个数:", digit_count)
print("其他字符个数:", other_count)
```
你可以将任意字符串输入到代码中,并获得结果。请注意,代码中的字符串变量只接受单行输入,如果想要输入多行字符,请使用循环或其他技巧来分割字符串并统计每个部分的字符个数。
阅读全文
相关推荐
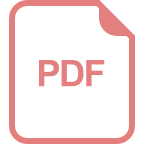
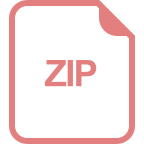












