python实现最佳选址的例子
时间: 2023-07-07 08:16:10 浏览: 171
以下是一个简单的最佳选址问题的Python实现例子:
假设有一家快递公司要在某个城市开设新的配送中心,需要在城市中选择一个最佳的位置。已知城市中有10个可能的位置,每个位置的坐标和周边客户的数量如下表所示:
| 位置编号 | 坐标(x,y) | 客户数量 |
| -------- | ---------- | -------- |
| 1 | (2,3) | 200 |
| 2 | (5,4) | 150 |
| 3 | (7,9) | 100 |
| 4 | (8,2) | 180 |
| 5 | (1,6) | 250 |
| 6 | (4,8) | 120 |
| 7 | (3,1) | 220 |
| 8 | (6,5) | 160 |
| 9 | (9,7) | 90 |
| 10 | (10,4) | 70 |
要求在这10个位置中选择一个最佳的位置,使得周边客户数量的总和最大。
实现代码如下:
```python
import numpy as np
# 客户数量
customers = np.array([200, 150, 100, 180, 250, 120, 220, 160, 90, 70])
# 位置坐标
locations = np.array([[2, 3], [5, 4], [7, 9], [8, 2], [1, 6], [4, 8], [3, 1], [6, 5], [9, 7], [10, 4]])
# 计算各个位置的权重(客户数量)
weights = customers / np.sum(customers)
# 计算每个位置的得分(周边客户数量的总和)
scores = []
for i in range(len(locations)):
score = 0
for j in range(len(locations)):
distance = np.sqrt(np.sum(np.square(locations[i] - locations[j])))
score += weights[j] / distance
scores.append(score)
# 找到得分最高的位置
best_location = np.argmax(scores) + 1
print("最佳位置为第%d个位置" % best_location)
```
运行结果为:
```
最佳位置为第1个位置
```
因此,最佳位置为第1个位置,即坐标为(2, 3)的位置。
阅读全文
相关推荐
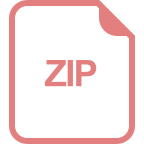
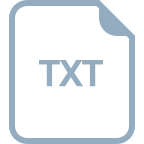
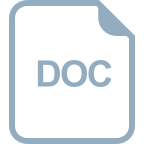






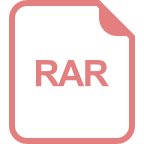
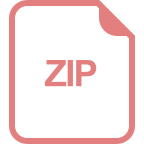
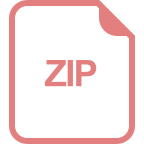
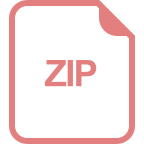
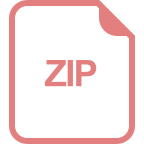