用C语言写一个给时间的类定义构造函数,要求包括小时,分钟,秒,用户名,函数需运行时间的初始化,销毁,设置,显示
时间: 2024-05-27 17:14:11 浏览: 34
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 时间类的定义
typedef struct Time {
int hour;
int minute;
int second;
char* username;
} Time;
// 构造函数
Time* create_time(int hour, int minute, int second, char* username) {
Time* t = (Time*) malloc(sizeof(Time));
t->hour = hour;
t->minute = minute;
t->second = second;
t->username = (char*) malloc(sizeof(char) * (strlen(username) + 1));
strcpy(t->username, username);
return t;
}
// 销毁函数
void destroy_time(Time* t) {
free(t->username);
free(t);
}
// 设置函数
void set_time(Time* t, int hour, int minute, int second, char* username) {
t->hour = hour;
t->minute = minute;
t->second = second;
if (strlen(t->username) < strlen(username)) {
t->username = (char*) realloc(t->username, sizeof(char) * (strlen(username) + 1));
}
strcpy(t->username, username);
}
// 显示函数
void show_time(Time* t) {
printf("%02d:%02d:%02d by %s\n", t->hour, t->minute, t->second, t->username);
}
int main() {
// 创建时间对象
Time* t = create_time(10, 30, 45, "Tom");
// 显示时间
show_time(t);
// 修改时间和用户名
set_time(t, 15, 20, 35, "Jerry");
// 显示修改后的时间
show_time(t);
// 销毁时间对象
destroy_time(t);
return 0;
}
阅读全文
相关推荐
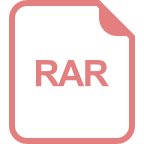

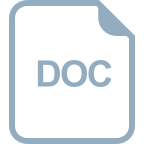


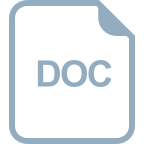
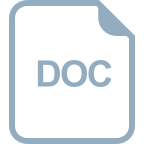
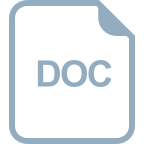
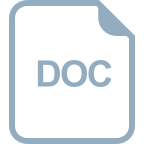
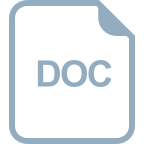
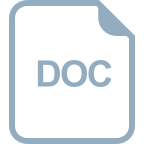




