解释代码deftrain(): device_ids=[int(i)foriinargs.gpus.split(',')]
时间: 2024-05-22 20:10:39 浏览: 125
这段代码是定义一个函数 `deftrain()`,其中含有一个参数 `args`。在这里,我们使用了 `args.gpus.split(',')`,将 `args.gpus` 以逗号为分隔符进行分割,得到一个由字符串组成的列表。然后,我们使用了列表推导式 `[int(i) for i in args.gpus.split(',')]`,将列表中的每个字符串转换为整数类型,并将其存储在一个新的列表 `device_ids` 中。最后,这个新的列表 `device_ids` 将被用作 PyTorch 的 `DataParallel` 模块的参数,用于指定在哪些 GPU 上运行模型训练。
相关问题
怎么加快这段代码的运行速度num_samples = x_data.shape[0] features = tf.Variable(initial_value=tf.zeros((1, 0)), dtype=tf.float32) batch_size = 32 for i in range(0, num_samples, batch_size): batch = x_data[i:i + batch_size] batch = tf.expand_dims(batch, axis=0) if i + batch_size > num_samples: batch_num = num_samples - i if batch_num == 1: feature_batch = model.predict(batch.reshape(1, *input_shape), batch_size=batch_num) else: feature_batch = model.predict(batch, batch_size=batch_num) features = tf.concat([features, feature_batch], axis=1) else: feature_batch = model.predict(batch, batch_size=batch_size) features = tf.concat([features, feature_batch], axis=1) print(features.shape)
这段代码的运行速度可以通过以下几种方式进行优化:
1. 使用更快的模型:如果模型的计算速度较慢,可以尝试使用更快的模型,例如MobileNet、EfficientNet等。
2. 使用GPU加速:如果你的电脑有GPU,可以使用GPU加速模型的计算。可以使用`tf.config.experimental.set_memory_growth()`方法来设置GPU内存分配策略,以避免内存溢出问题。
3. 使用TensorFlow Dataset:TensorFlow Dataset是一种高效的数据输入管道,可以帮助提高训练速度。可以使用`tf.data.Dataset.from_tensor_slices()`方法来创建一个Dataset对象,然后使用`batch()`方法和`prefetch()`方法来设置批量大小和预取数据。
4. 使用更大的批量大小:如果你的电脑有足够的内存,可以尝试使用更大的批量大小,以提高训练速度。但是需要注意,批量大小过大可能会导致内存溢出问题。
5. 使用多线程预处理数据:如果你的电脑有多个CPU核心,可以使用多线程预处理数据,以加快数据处理速度。可以使用`tf.data.Dataset.map()`方法来定义一个数据预处理函数,并使用`num_parallel_calls`参数来设置线程数。
根据你的代码,可以使用方法1、2和4来进行优化,具体代码如下:
```python
# 方法1:使用更快的模型
from tensorflow.keras.applications import MobileNetV2
model = MobileNetV2(input_shape=input_shape, include_top=False, weights='imagenet')
# 方法2:使用GPU加速
gpus = tf.config.list_physical_devices('GPU')
if gpus:
try:
tf.config.experimental.set_memory_growth(gpus[0], True)
except RuntimeError as e:
print(e)
# 方法4:使用更大的批量大小
batch_size = 64
# 修改后的代码
features = tf.Variable(initial_value=tf.zeros((1, 0)), dtype=tf.float32)
for i in range(0, num_samples, batch_size):
batch = x_data[i:i + batch_size]
batch = tf.expand_dims(batch, axis=0)
if i + batch_size > num_samples:
batch_num = num_samples - i
if batch_num == 1:
feature_batch = model.predict(batch.reshape(1, *input_shape), batch_size=batch_num)
else:
feature_batch = model.predict(batch, batch_size=batch_num)
features = tf.concat([features, feature_batch], axis=1)
else:
feature_batch = model.predict(batch, batch_size=batch_size)
features = tf.concat([features, feature_batch], axis=1)
print(features.shape)
```
希望这些方法能够帮助你加快代码的运行速度!
processor_cfg: type: "processor.pose_demo.inference" gpus: 1 worker_per_gpu: 1 video_file: resource/data_example/skateboarding.mp4 save_dir: "work_dir/pose_demo" detection_cfg: model_cfg: configs/mmdet/cascade_rcnn_r50_fpn_1x.py checkpoint_file: mmskeleton://mmdet/cascade_rcnn_r50_fpn_20e bbox_thre: 0.8 estimation_cfg: model_cfg: configs/pose_estimation/hrnet/pose_hrnet_w32_256x192_test.yaml checkpoint_file: mmskeleton://pose_estimation/pose_hrnet_w32_256x192 data_cfg: image_size: - 192 - 256 pixel_std: 200 image_mean: - 0.485 - 0.456 - 0.406 image_std: - 0.229 - 0.224 - 0.225 post_process: true argparse_cfg: gpus: bind_to: processor_cfg.gpus help: number of gpus video: bind_to: processor_cfg.video_file help: path to input video worker_per_gpu: bind_to: processor_cfg.worker_per_gpu help: number of workers for each gpu skeleton_model: bind_to: processor_cfg.estimation_cfg.model_cfg skeleton_checkpoint: bind_to: processor_cfg.estimation_cfg.checkpoint_file detection_model: bind_to: processor_cfg.detection_cfg.model_cfg detection_checkpoint: bind_to: processor_cfg.detection_cfg.checkpoint_file
根据您提供的配置文件,这是一个用于姿态估计的pose_demo的配置示例。该配置文件包括了处理器配置(processor_cfg)和命令行参数配置(argparse_cfg)。
处理器配置包括以下内容:
- type:指定处理器类型为"processor.pose_demo.inference",这可能是一个自定义的处理器类型。
- gpus:指定使用的GPU数量为1。
- worker_per_gpu:指定每个GPU的worker数量为1。
- video_file:指定输入视频的路径为"resource/data_example/skateboarding.mp4"。
- save_dir:指定结果保存的目录路径为"work_dir/pose_demo"。
检测配置(detection_cfg)包括以下内容:
- model_cfg:指定检测模型的配置文件路径为"configs/mmdet/cascade_rcnn_r50_fpn_1x.py"。
- checkpoint_file:指定检测模型的checkpoint路径为"mmskeleton://mmdet/cascade_rcnn_r50_fpn_20e"。
- bbox_thre:指定检测目标的边界框阈值为0.8。
估计配置(estimation_cfg)包括以下内容:
- model_cfg:指定姿态估计模型的配置文件路径为"configs/pose_estimation/hrnet/pose_hrnet_w32_256x192_test.yaml"。
- checkpoint_file:指定姿态估计模型的checkpoint路径为"mmskeleton://pose_estimation/pose_hrnet_w32_256x192"。
- data_cfg:指定姿态估计模型的数据配置,包括图像尺寸、像素标准化值、图像均值和标准差以及后处理选项。
命令行参数配置(argparse_cfg)包括了一些命令行参数的绑定,用于从命令行传递参数给处理器配置。
例如,您可以使用以下命令行参数来运行pose_demo:
```
python pose_demo.py --gpus 1 --video resource/data_example/skateboarding.mp4 --worker_per_gpu 1 --skeleton_model configs/pose_estimation/hrnet/pose_hrnet_w32_256x192_test.yaml --skeleton_checkpoint mmskeleton://pose_estimation/pose_hrnet_w32_256x192 --detection_model configs/mmdet/cascade_rcnn_r50_fpn_1x.py --detection_checkpoint mmskeleton://mmdet/cascade_rcnn_r50_fpn_20e
```
请注意,以上仅为示例,具体的使用方式和命令行参数可能会有所不同,具体取决于实际情况。
如果您有进一步的问题,请提供更多细节,我将尽力帮助您。
阅读全文
相关推荐
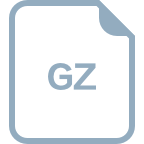
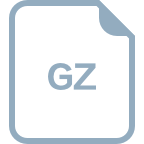
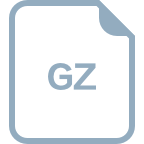












