编写 SPI串口屏python驱动代码
时间: 2024-04-19 14:07:36 浏览: 121
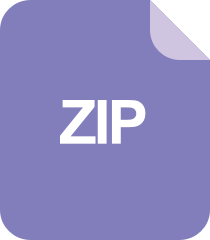
USB2SPI_ADS1256Test_python_

以下是一个简单的SPI串口屏python驱动代码,可以根据实际情况进行修改和优化:
```python
import spidev
import time
class SPI_Screen_Driver:
def __init__(self, spi_port=0, spi_device=0):
self.spi = spidev.SpiDev()
self.spi.open(spi_port, spi_device)
self.spi.max_speed_hz = 1000000 # 设置SPI通信速度
self.spi.mode = 0b00 # 设置SPI通信模式
self.width = 128 # 屏幕宽度
self.height = 160 # 屏幕高度
def write_command(self, command):
self.spi.xfer2([0x70, command]) # 发送命令
def write_data(self, data):
self.spi.xfer2([0x72, data]) # 发送数据
def set_position(self, x, y):
self.write_command(0x2A)
self.write_data(0x00)
self.write_data(x+2)
self.write_data(0x00)
self.write_data(self.width-1)
self.write_command(0x2B)
self.write_data(0x00)
self.write_data(y+3)
self.write_data(0x00)
self.write_data(self.height-1)
self.write_command(0x2C)
def clear_screen(self):
self.set_position(0, 0)
for i in range(self.width * self.height):
self.write_data(0x00)
def draw_pixel(self, x, y, color):
if x >= self.width or y >= self.height:
return
self.set_position(x, y)
self.write_data((color >> 8) & 0xFF)
self.write_data(color & 0xFF)
def draw_line(self, x0, y0, x1, y1, color):
dx = abs(x1 - x0)
dy = abs(y1 - y0)
sx = -1 if x0 > x1 else 1
sy = -1 if y0 > y1 else 1
err = dx - dy
while True:
self.draw_pixel(x0, y0, color)
if x0 == x1 and y0 == y1:
break
e2 = 2 * err
if e2 > -dy:
err -= dy
x0 += sx
if e2 < dx:
err += dx
y0 += sy
def draw_rect(self, x, y, w, h, color):
for i in range(x, x + w):
for j in range(y, y + h):
if i == x or i == x + w - 1 or j == y or j == y + h - 1:
self.draw_pixel(i, j, color)
def draw_circle(self, x0, y0, r, color):
x = r
y = 0
err = 0
while x >= y:
self.draw_pixel(x0 + x, y0 + y, color)
self.draw_pixel(x0 + y, y0 + x, color)
self.draw_pixel(x0 - y, y0 + x, color)
self.draw_pixel(x0 - x, y0 + y, color)
self.draw_pixel(x0 - x, y0 - y, color)
self.draw_pixel(x0 - y, y0 - x, color)
self.draw_pixel(x0 + y, y0 - x, color)
self.draw_pixel(x0 + x, y0 - y, color)
if err <= 0:
y += 1
err += 2*y + 1
if err > 0:
x -= 1
err -= 2*x + 1
def display_image(self, image):
self.set_position(0, 0)
for i in range(self.width * self.height):
self.write_data(image[i])
```
这个代码中包含了一些基本的绘图函数,如绘制像素点、直线、矩形和圆形,以及显示图片的函数。你可以根据具体的需求,进一步完善和优化这个驱动代码。
阅读全文
相关推荐
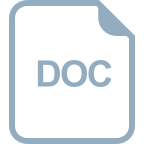
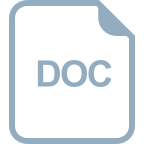
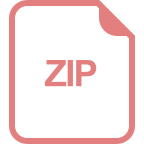
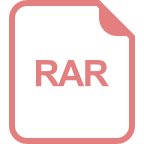
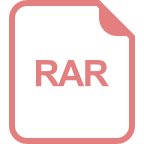
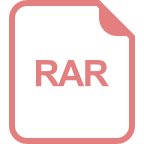
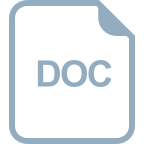
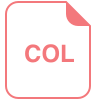
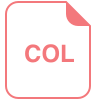
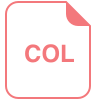







