两个有序链表的交集c语言实现
时间: 2023-07-12 14:34:35 浏览: 66
以下是两个有序链表的交集的C语言实现代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
struct ListNode {
int val;
struct ListNode *next;
};
// 定义函数,用于创建链表节点
struct ListNode* createListNode(int val) {
struct ListNode* node = (struct ListNode*)malloc(sizeof(struct ListNode));
node->val = val;
node->next = NULL;
return node;
}
// 定义函数,用于打印链表
void printList(struct ListNode* head) {
struct ListNode* p = head;
while(p != NULL) {
printf("%d ", p->val);
p = p->next;
}
printf("\n");
}
// 定义函数,用于获取两个有序链表的交集
struct ListNode* getIntersection(struct ListNode* head1, struct ListNode* head2) {
struct ListNode* p1 = head1;
struct ListNode* p2 = head2;
struct ListNode* head = NULL;
struct ListNode* tail = NULL;
while(p1 != NULL && p2 != NULL) {
if(p1->val == p2->val) {
struct ListNode* node = createListNode(p1->val);
if(head == NULL) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
p1 = p1->next;
p2 = p2->next;
} else if(p1->val < p2->val) {
p1 = p1->next;
} else {
p2 = p2->next;
}
}
return head;
}
int main() {
// 创建链表1: 1->2->4->5
struct ListNode* head1 = createListNode(1);
head1->next = createListNode(2);
head1->next->next = createListNode(4);
head1->next->next->next = createListNode(5);
printf("链表1: ");
printList(head1);
// 创建链表2: 2->3->5->6
struct ListNode* head2 = createListNode(2);
head2->next = createListNode(3);
head2->next->next = createListNode(5);
head2->next->next->next = createListNode(6);
printf("链表2: ");
printList(head2);
// 获取两个有序链表的交集
struct ListNode* intersection = getIntersection(head1, head2);
printf("交集: ");
printList(intersection);
return 0;
}
```
该代码首先定义了一个链表节点结构体,然后定义了三个函数:`createListNode()`用于创建链表节点,`printList()`用于打印链表,`getIntersection()`用于获取两个有序链表的交集。
在`getIntersection()`函数中,定义了两个指针`p1`和`p2`,分别指向两个链表的头结点。然后使用while循环遍历这两个链表,如果`p1->val`等于`p2->val`,就说明这个节点是交集中的元素,于是创建一个新的节点,并将其添加到交集链表中。如果`p1->val`小于`p2->val`,说明`p1`所指向的节点在`p2`所指向的节点之前,因此将`p1`向后移动一位。如果`p1->val`大于`p2->val`,说明`p2`所指向的节点在`p1`所指向的节点之前,因此将`p2`向后移动一位。最后返回交集链表的头结点。
在`main()`函数中,先创建了两个有序链表,然后调用`getIntersection()`函数获取它们的交集,并打印输出。
相关推荐
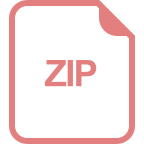
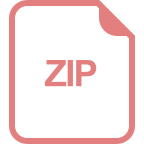










