c语言写利用有序链表表示下整数任命,实现信息的交、并和差运算。
时间: 2024-03-14 10:46:19 浏览: 64
下面是利用有序链表表示下整数任命,实现信息的交、并和差运算的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int data;
struct node *next;
} Node;
// 创建链表
Node *createList() {
Node *head = (Node *)malloc(sizeof(Node));
head->next = NULL;
return head;
}
// 插入元素
void insert(Node *head, int data) {
Node *p = head;
while (p->next != NULL && p->next->data < data) {
p = p->next;
}
Node *newNode = (Node *)malloc(sizeof(Node));
newNode->data = data;
newNode->next = p->next;
p->next = newNode;
}
// 交集运算
Node *intersection(Node *list1, Node *list2) {
Node *result = createList();
Node *p1 = list1->next;
Node *p2 = list2->next;
while (p1 != NULL && p2 != NULL) {
if (p1->data == p2->data) {
insert(result, p1->data);
p1 = p1->next;
p2 = p2->next;
} else if (p1->data < p2->data) {
p1 = p1->next;
} else {
p2 = p2->next;
}
}
return result;
}
// 并集运算
Node *unionSet(Node *list1, Node *list2) {
Node *result = createList();
Node *p1 = list1->next;
Node *p2 = list2->next;
while (p1 != NULL && p2 != NULL) {
if (p1->data == p2->data) {
insert(result, p1->data);
p1 = p1->next;
p2 = p2->next;
} else if (p1->data < p2->data) {
insert(result, p1->data);
p1 = p1->next;
} else {
insert(result, p2->data);
p2 = p2->next;
}
}
while (p1 != NULL) {
insert(result, p1->data);
p1 = p1->next;
}
while (p2 != NULL) {
insert(result, p2->data);
p2 = p2->next;
}
return result;
}
// 差集运算
Node *difference(Node *list1, Node *list2) {
Node *result = createList();
Node *p1 = list1->next;
Node *p2 = list2->next;
while (p1 != NULL && p2 != NULL) {
if (p1->data == p2->data) {
p1 = p1->next;
p2 = p2->next;
} else if (p1->data < p2->data) {
insert(result, p1->data);
p1 = p1->next;
} else {
p2 = p2->next;
}
}
while (p1 != NULL) {
insert(result, p1->data);
p1 = p1->next;
}
return result;
}
// 打印链表
void printList(Node *list) {
Node *p = list->next;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
int main() {
Node *list1 = createList();
Node *list2 = createList();
insert(list1, 1);
insert(list1, 3);
insert(list1, 5);
insert(list2, 2);
insert(list2, 3);
insert(list2, 4);
printf("list1: ");
printList(list1);
printf("list2: ");
printList(list2);
printf("intersection: ");
printList(intersection(list1, list2));
printf("union: ");
printList(unionSet(list1, list2));
printf("difference: ");
printList(difference(list1, list2));
return 0;
}
```
代码的思路是先创建两个有序链表,然后实现插入元素、交集运算、并集运算和差集运算等基本操作。具体实现见代码注释。
阅读全文
相关推荐
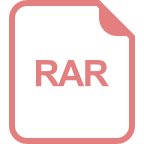
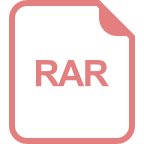
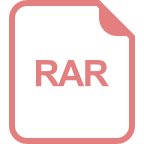
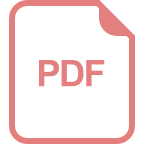
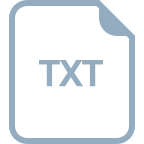
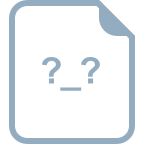
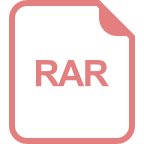
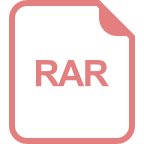