java dictionary遍历_遍历Dictionary<K,V>的两种方式
时间: 2024-01-19 18:40:44 浏览: 53
Dictionary<K,V>是一个抽象类,它定义了一个键值对的映射表。它有两个主要的实现类:Hashtable和Properties。
遍历Dictionary<K,V>有两种方式:
1. 迭代器遍历
使用迭代器遍历Dictionary<K,V>,需要先通过keys()方法获取Enumeration<K>类型的键的枚举集合,然后通过while循环遍历枚举集合,获取每一个键,并通过get()方法获取对应的值。
示例代码:
```java
Dictionary<String, Integer> dict = new Hashtable<>();
dict.put("a", 1);
dict.put("b", 2);
dict.put("c", 3);
Enumeration<String> keys = dict.keys();
while (keys.hasMoreElements()) {
String key = keys.nextElement();
Integer value = dict.get(key);
System.out.println(key + ": " + value);
}
```
输出结果:
```
a: 1
b: 2
c: 3
```
2. foreach遍历
从Java 8开始,Java引入了foreach循环,可以直接遍历集合对象。由于Dictionary<K,V>没有实现Iterable接口,因此需要通过Collections.list()方法将枚举集合转换为一个List集合,然后通过foreach循环遍历List集合,获取每一个键值对。
示例代码:
```java
Dictionary<String, Integer> dict = new Hashtable<>();
dict.put("a", 1);
dict.put("b", 2);
dict.put("c", 3);
List<Map.Entry<String, Integer>> list = Collections.list(dict.entries());
for (Map.Entry<String, Integer> entry : list) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
```
输出结果:
```
a: 1
b: 2
c: 3
```
相关推荐
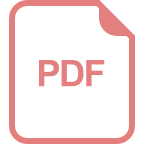
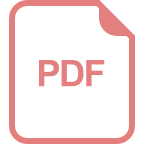














