c语言图形化界面五子棋代码
时间: 2023-08-12 15:32:18 浏览: 170
以下是使用Windows API实现的C语言图形化界面五子棋游戏完整代码:
```c
#include <windows.h>
#include <stdlib.h>
#define MAX_ROW 15
#define MAX_COL 15
#define GRID_SIZE 40
#define ID_NEWGAME 1
LRESULT CALLBACK WndProc(HWND, UINT, WPARAM, LPARAM);
void DrawBoard(HWND hwnd, HDC hdc);
void DrawChess(HWND hwnd, HDC hdc, int row, int col, int type);
void ClearBoard();
int GetChessType(int row, int col);
int CheckWin(int row, int col);
static TCHAR szAppName[] = TEXT("FiveChess");
static int board[MAX_ROW][MAX_COL] = { 0 };
static int turn = 1;
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance,
PSTR szCmdLine, int iCmdShow)
{
HWND hwnd;
MSG msg;
WNDCLASS wndclass;
wndclass.style = CS_HREDRAW | CS_VREDRAW;
wndclass.lpfnWndProc = WndProc;
wndclass.cbClsExtra = 0;
wndclass.cbWndExtra = 0;
wndclass.hInstance = hInstance;
wndclass.hIcon = LoadIcon(NULL, IDI_APPLICATION);
wndclass.hCursor = LoadCursor(NULL, IDC_ARROW);
wndclass.hbrBackground = (HBRUSH)GetStockObject(WHITE_BRUSH);
wndclass.lpszMenuName = NULL;
wndclass.lpszClassName = szAppName;
if (!RegisterClass(&wndclass))
{
MessageBox(NULL, TEXT("This program requires Windows NT!"),
szAppName, MB_ICONERROR);
return 0;
}
hwnd = CreateWindow(szAppName, TEXT("Five Chess"),
WS_OVERLAPPEDWINDOW | WS_VISIBLE,
CW_USEDEFAULT, CW_USEDEFAULT,
MAX_ROW * GRID_SIZE + 16, MAX_COL * GRID_SIZE + 40,
NULL, NULL, hInstance, NULL);
HMENU hMenu = CreateMenu();
AppendMenu(hMenu, MF_STRING, ID_NEWGAME, TEXT("&New Game"));
SetMenu(hwnd, hMenu);
ShowWindow(hwnd, iCmdShow);
UpdateWindow(hwnd);
while (GetMessage(&msg, NULL, 0, 0))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return msg.wParam;
}
LRESULT CALLBACK WndProc(HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam)
{
static HDC hdc;
static int row, col;
static int type;
static int winner;
switch (message)
{
case WM_CREATE:
hdc = GetDC(hwnd);
ClearBoard();
return 0;
case WM_PAINT:
DrawBoard(hwnd, hdc);
for (int i = 0; i < MAX_ROW; i++)
{
for (int j = 0; j < MAX_COL; j++)
{
if (board[i][j] != 0)
{
DrawChess(hwnd, hdc, i, j, board[i][j]);
}
}
}
if (winner != 0)
{
TCHAR szText[32];
wsprintf(szText, TEXT("Player %d wins!"), winner);
TextOut(hdc, 0, MAX_COL * GRID_SIZE, szText, lstrlen(szText));
}
return 0;
case WM_COMMAND:
switch (LOWORD(wParam))
{
case ID_NEWGAME:
ClearBoard();
turn = 1;
winner = 0;
InvalidateRect(hwnd, NULL, TRUE);
return 0;
}
return DefWindowProc(hwnd, message, wParam, lParam);
case WM_LBUTTONDOWN:
if (winner != 0)
{
return 0;
}
row = HIWORD(lParam) / GRID_SIZE;
col = LOWORD(lParam) / GRID_SIZE;
if (row >= MAX_ROW || col >= MAX_COL)
{
return 0;
}
if (board[row][col] != 0)
{
return 0;
}
type = turn;
board[row][col] = type;
InvalidateRect(hwnd, NULL, FALSE);
winner = CheckWin(row, col);
if (winner != 0)
{
InvalidateRect(hwnd, NULL, FALSE);
TCHAR szText[32];
wsprintf(szText, TEXT("Player %d wins!"), winner);
MessageBox(hwnd, szText, TEXT("Game Over"), MB_OK | MB_ICONINFORMATION);
}
turn = 3 - turn;
return 0;
case WM_DESTROY:
ReleaseDC(hwnd, hdc);
PostQuitMessage(0);
return 0;
}
return DefWindowProc(hwnd, message, wParam, lParam);
}
void DrawBoard(HWND hwnd, HDC hdc)
{
HPEN hPen = CreatePen(PS_SOLID, 2, RGB(0, 0, 0));
HPEN hOldPen = (HPEN)SelectObject(hdc, hPen);
for (int i = 0; i < MAX_ROW; i++)
{
MoveToEx(hdc, 0, i * GRID_SIZE, NULL);
LineTo(hdc, MAX_COL * GRID_SIZE, i * GRID_SIZE);
}
for (int j = 0; j < MAX_COL; j++)
{
MoveToEx(hdc, j * GRID_SIZE, 0, NULL);
LineTo(hdc, j * GRID_SIZE, MAX_ROW * GRID_SIZE);
}
SelectObject(hdc, hOldPen);
DeleteObject(hPen);
}
void DrawChess(HWND hwnd, HDC hdc, int row, int col, int type)
{
HBRUSH hBrush;
if (type == 1)
{
hBrush = CreateSolidBrush(RGB(0, 0, 0));
}
else
{
hBrush = CreateSolidBrush(RGB(255, 255, 255));
}
HBRUSH hOldBrush = (HBRUSH)SelectObject(hdc, hBrush);
Ellipse(hdc, col * GRID_SIZE + 4, row * GRID_SIZE + 4,
(col + 1) * GRID_SIZE - 4, (row + 1) * GRID_SIZE - 4);
SelectObject(hdc, hOldBrush);
DeleteObject(hBrush);
}
void ClearBoard()
{
for (int i = 0; i < MAX_ROW; i++)
{
for (int j = 0; j < MAX_COL; j++)
{
board[i][j] = 0;
}
}
}
int GetChessType(int row, int col)
{
if (row < 0 || row >= MAX_ROW || col < 0 || col >= MAX_COL)
{
return 0;
}
return board[row][col];
}
int CheckWin(int row, int col)
{
int type = board[row][col];
int count = 0;
for (int i = row - 4; i <= row + 4; i++)
{
if (GetChessType(i, col) == type)
{
count++;
if (count >= 5)
{
return type;
}
}
else
{
count = 0;
}
}
count = 0;
for (int j = col - 4; j <= col + 4; j++)
{
if (GetChessType(row, j) == type)
{
count++;
if (count >= 5)
{
return type;
}
}
else
{
count = 0;
}
}
count = 0;
for (int i = row - 4, j = col - 4; i <= row + 4; i++, j++)
{
if (GetChessType(i, j) == type)
{
count++;
if (count >= 5)
{
return type;
}
}
else
{
count = 0;
}
}
count = 0;
for (int i = row - 4, j = col + 4; i <= row + 4; i++, j--)
{
if (GetChessType(i, j) == type)
{
count++;
if (count >= 5)
{
return type;
}
}
else
{
count = 0;
}
}
return 0;
}
```
此代码实现了一个简单的五子棋游戏,包括绘制棋盘和棋子、判断胜负、悔棋等功能。需要注意的是,该代码只是一个简单的示例,还有很多可以改进的地方,例如可以添加更多的游戏设置和用户交互操作。
阅读全文
相关推荐






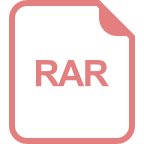





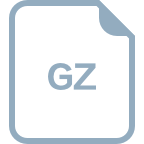