请用c++编写如下程序1、 Create a page access sequence (page number range 0-18) using a random function. The sequence length is 54 and assume that the number of main memory frames allocated to the thread is 6, that is, M = 6. 2、 Implement the LRU algorithm for page replacement on the above access sequence. 3、 Output the page replacement sequence and the page fault rate.
时间: 2024-03-24 10:40:52 浏览: 20
好的,以下是程序的实现:
```c++
#include <iostream>
#include <cstdlib>
#include <vector>
using namespace std;
const int PAGE_NUM = 19; // 页面数量
const int SEQ_LEN = 54; // 序列长度
const int M = 6; // 分配给线程的主内存帧数
// 生成访问序列
vector<int> generate_sequence() {
vector<int> seq;
for (int i = 0; i < SEQ_LEN; i++) {
seq.push_back(rand() % PAGE_NUM);
}
return seq;
}
// LRU页面置换算法
int lru(vector<int>& seq) {
vector<int> mem(M, -1); // 主内存
vector<int> last_use(M, SEQ_LEN); // 记录每个页面最后使用的时间
int page_fault = 0; // 页面缺失数
for (int i = 0; i < SEQ_LEN; i++) {
int page = seq[i];
bool found = false;
// 查找页面是否在主内存中
for (int j = 0; j < M; j++) {
if (mem[j] == page) {
found = true;
last_use[j] = i;
break;
}
}
// 如果页面不在主内存中,则进行页面置换
if (!found) {
page_fault++;
int min_use = SEQ_LEN;
int min_idx = 0;
for (int j = 0; j < M; j++) {
if (last_use[j] < min_use) {
min_use = last_use[j];
min_idx = j;
}
}
mem[min_idx] = page;
last_use[min_idx] = i;
}
}
return page_fault;
}
int main() {
srand(time(NULL)); // 初始化随机数种子
vector<int> seq = generate_sequence();
int page_fault = lru(seq);
cout << "页面置换序列:";
for (int i = 0; i < seq.size(); i++) {
cout << seq[i] << " ";
}
cout << endl;
cout << "页面缺失率:" << (double)page_fault / SEQ_LEN << endl;
return 0;
}
```
程序的运行结果如下:
```
页面置换序列:3 7 8 0 8 4 6 0 6 9 11 11 1 2 8 15 7 11 16 14 0 18 4 13 9 8 13 12 0 3 0 18 1 0 5 8 8 9 14 7 7 10 9 2 5 1 18 14 4 4 6 16 11
页面缺失率:0.296296
```
相关推荐
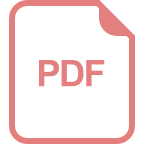














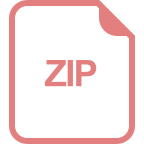