基于谱减法的自适应消噪算法C语言
时间: 2023-09-11 10:07:00 浏览: 78
以下是一个基于谱减法的自适应消噪算法的C语言示例:
```c
#define FRAME_SIZE 256
#define OVERLAP_SIZE 128
#define THRESHOLD 30
float noise_spectrum[FRAME_SIZE] = {0};
float alpha = 0.1;
void subtract_noise(float* input_frame, float* output_frame)
{
float spectrum[FRAME_SIZE] = {0};
float magnitude[FRAME_SIZE] = {0};
float phase[FRAME_SIZE] = {0};
float noise_magnitude[FRAME_SIZE] = {0};
float ratio[FRAME_SIZE] = {0};
float output_spectrum[FRAME_SIZE] = {0};
float output_frame_temp[FRAME_SIZE] = {0};
int i;
// Calculate the magnitude and phase of input frame using FFT
fft(input_frame, spectrum, phase, FRAME_SIZE);
// Calculate the magnitude of noise spectrum using exponential smoothing
for (i = 0; i < FRAME_SIZE; i++)
{
noise_spectrum[i] = alpha * noise_spectrum[i] + (1 - alpha) * spectrum[i];
noise_magnitude[i] = sqrtf(noise_spectrum[i]);
}
// Calculate the magnitude of input frame
for (i = 0; i < FRAME_SIZE; i++)
{
magnitude[i] = sqrtf(spectrum[i]);
}
// Calculate the ratio of magnitude of input frame and noise spectrum
for (i = 0; i < FRAME_SIZE; i++)
{
if (magnitude[i] > noise_magnitude[i])
{
ratio[i] = 20 * log10f(magnitude[i] / noise_magnitude[i]);
}
else
{
ratio[i] = 0;
}
}
// Subtract the noise floor
for (i = 0; i < FRAME_SIZE; i++)
{
if (ratio[i] < THRESHOLD)
{
output_spectrum[i] = noise_spectrum[i];
}
else
{
output_spectrum[i] = spectrum[i] - noise_spectrum[i];
}
}
// Calculate the output frame using IFFT
ifft(output_spectrum, phase, output_frame_temp, FRAME_SIZE);
// Overlap and add the output frame to the previous frame
for (i = 0; i < FRAME_SIZE; i++)
{
output_frame[i] = output_frame_temp[i] + input_frame[i + OVERLAP_SIZE];
}
// Shift the frames
for (i = 0; i < OVERLAP_SIZE; i++)
{
input_frame[i] = input_frame[i + OVERLAP_SIZE];
}
}
```
这个示例中,使用了一个长度为256的帧和一个长度为128的重叠。alpha为指数平滑系数,用于计算噪声谱。THRESHOLD为信噪比的阈值,低于该阈值的幅度被认为是噪声,而高于该阈值的幅度被认为是信号。
在subtract_noise函数中,首先使用FFT计算输入帧的幅度和相位谱,然后使用指数平滑法计算噪声谱,并计算输入帧幅度和噪声幅度的比值。根据信噪比阈值,将低于阈值的幅度视为噪声,而高于阈值的幅度视为信号,并将噪声谱从输入帧的幅度谱中减去,得到输出帧的幅度谱。最后使用IFFT计算输出帧,将其与前一个帧进行重叠和相加,得到最终的输出帧。
需要注意的是,该算法需要实时计算FFT和IFFT,因此在实际应用中需要考虑到性能和时间延迟等问题。
相关推荐
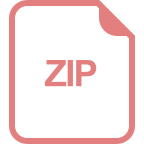
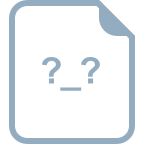














