python取出一个文件夹下所有excel文件中sheet_name=“系数表”的内容,将内容行列转置,全部合并后生成EXCEL后导出;
时间: 2024-03-25 22:42:02 浏览: 14
好的,我来为您解答。
实现这个功能需要用到Python中的pandas库和openpyxl库。具体步骤如下:
1. 导入所需库
```python
import os
import pandas as pd
from openpyxl import Workbook
```
2. 定义函数,用于获取文件夹下所有excel文件中sheet_name=“系数表”的内容,并将内容行列转置
```python
def get_excel_data(file_path):
df_list = []
for file_name in os.listdir(file_path):
if file_name.endswith('.xlsx'):
file = os.path.join(file_path, file_name)
excel_data = pd.read_excel(file, sheet_name='系数表')
excel_data_T = excel_data.T
df_list.append(excel_data_T)
return pd.concat(df_list)
```
3. 调用函数,将获取到的所有excel文件中sheet_name=“系数表”的内容全部合并
```python
df = get_excel_data('your_file_path')
```
4. 创建一个Workbook对象,将合并后的内容写入Excel文件中
```python
wb = Workbook()
ws = wb.active
for r in dataframe_to_rows(df, index=True, header=True):
ws.append(r)
wb.save('output.xlsx')
```
完整代码如下:
```python
import os
import pandas as pd
from openpyxl import Workbook
from openpyxl.utils.dataframe import dataframe_to_rows
def get_excel_data(file_path):
df_list = []
for file_name in os.listdir(file_path):
if file_name.endswith('.xlsx'):
file = os.path.join(file_path, file_name)
excel_data = pd.read_excel(file, sheet_name='系数表')
excel_data_T = excel_data.T
df_list.append(excel_data_T)
return pd.concat(df_list)
df = get_excel_data('your_file_path')
wb = Workbook()
ws = wb.active
for r in dataframe_to_rows(df, index=True, header=True):
ws.append(r)
wb.save('output.xlsx')
```
将代码中的'your_file_path'替换成您需要处理的文件夹的路径,运行程序即可生成一个Excel文件,其中包含了所有excel文件中sheet_name=“系数表”的内容,并已经行列转置和合并。
相关推荐
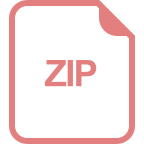









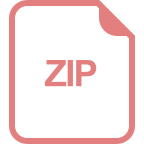