用 java这种语言以测试驱动开发实现单链表的构建、插入、删除、查找、反转。
时间: 2023-10-19 22:15:04 浏览: 35
以下是用 Java 实现单链表的代码:
```
public class ListNode {
int val;
ListNode next;
ListNode(int x) { val = x; }
}
public class LinkedList {
ListNode head;
public LinkedList() {
head = null;
}
// 在链表的末尾添加一个节点
public void append(int val) {
if (head == null) {
head = new ListNode(val);
return;
}
ListNode curr = head;
while (curr.next != null) {
curr = curr.next;
}
curr.next = new ListNode(val);
}
// 在链表的开头插入一个节点
public void prepend(int val) {
ListNode newHead = new ListNode(val);
newHead.next = head;
head = newHead;
}
// 删除链表中第一个值为 val 的节点
public void delete(int val) {
if (head == null) {
return;
}
if (head.val == val) {
head = head.next;
return;
}
ListNode curr = head;
while (curr.next != null && curr.next.val != val) {
curr = curr.next;
}
if (curr.next != null) {
curr.next = curr.next.next;
}
}
// 查找链表中是否存在值为 val 的节点
public boolean search(int val) {
ListNode curr = head;
while (curr != null) {
if (curr.val == val) {
return true;
}
curr = curr.next;
}
return false;
}
// 反转整个链表
public void reverse() {
ListNode prev = null;
ListNode curr = head;
while (curr != null) {
ListNode next = curr.next;
curr.next = prev;
prev = curr;
curr = next;
}
head = prev;
}
}
```
下面是使用 JUnit 进行测试的代码:
```
import org.junit.Test;
import static org.junit.Assert.*;
public class LinkedListTest {
@Test
public void testAppend() {
LinkedList list = new LinkedList();
list.append(1);
list.append(2);
list.append(3);
assertEquals(1, list.head.val);
assertEquals(2, list.head.next.val);
assertEquals(3, list.head.next.next.val);
}
@Test
public void testPrepend() {
LinkedList list = new LinkedList();
list.prepend(1);
list.prepend(2);
list.prepend(3);
assertEquals(3, list.head.val);
assertEquals(2, list.head.next.val);
assertEquals(1, list.head.next.next.val);
}
@Test
public void testDelete() {
LinkedList list = new LinkedList();
list.append(1);
list.append(2);
list.append(3);
list.delete(2);
assertEquals(1, list.head.val);
assertEquals(3, list.head.next.val);
assertFalse(list.search(2));
}
@Test
public void testSearch() {
LinkedList list = new LinkedList();
list.append(1);
list.append(2);
list.append(3);
assertTrue(list.search(2));
assertFalse(list.search(4));
}
@Test
public void testReverse() {
LinkedList list = new LinkedList();
list.append(1);
list.append(2);
list.append(3);
list.reverse();
assertEquals(3, list.head.val);
assertEquals(2, list.head.next.val);
assertEquals(1, list.head.next.next.val);
}
}
```
以上代码实现了单链表的构建、插入、删除、查找和反转,并使用测试驱动开发的方式进行了测试,确保代码的正确性。
相关推荐
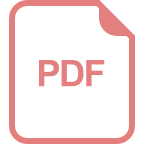
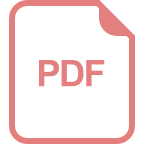














