c++ std::getline()函数的用法
时间: 2023-12-15 21:03:30 浏览: 95
`std::getline()` 是 C++ 标准库中的一个函数,用于从给定的输入流中读取一行数据。
其函数原型如下:
```c++
std::istream& getline(std::istream& is, std::string& str, char delim);
```
其中:
- `is`:输入流对象
- `str`:存储读取数据的字符串对象
- `delim`:指定分隔符,可选参数,默认为 `'\n'`
该函数会从输入流中读取字符,直到遇到指定分隔符或者文件结束符,然后将读取的字符存储到 `str` 中,并且不包括分隔符。如果指定分隔符为文件结束符,则读取到文件结束位置。
函数返回输入流对象的引用,可以用于连续读取多个行数据。
示例代码:
```c++
#include <iostream>
#include <string>
int main() {
std::string line;
std::cout << "Please enter a line of text: ";
std::getline(std::cin, line);
std::cout << "You entered: " << line << std::endl;
return 0;
}
```
运行结果:
```
Please enter a line of text: Hello, world!
You entered: Hello, world!
```
相关问题
std::getline
`std::getline` 是 C++ 中的一个函数,用于从输入流中读取一行字符串并存储到指定的字符串变量中。它的定义如下:
```cpp
std::istream& std::getline(std::istream& is, std::string& str, char delim);
```
其中,`is` 是输入流对象,`str` 是要存储读取到的字符串的变量,`delim` 是分隔符,表示输入流中一行字符串的结束位置,可以是换行符、回车符等任何字符。当读取到分隔符时,`std::getline` 会停止读取,将读取到的字符串存储到 `str` 中,并将分隔符从输入流中去掉。`std::getline` 函数返回输入流对象 `is` 的引用,以便进行链式操作。
std::getline(std::cin,line)
`std::getline(std::cin, line)`是C++中的一个函数,用于从标准输入流`std::cin`中读取一行文本,并将其存储在字符串变量`line`中。这个函数可以用来读取用户输入的一行文本。
以下是一个示例代码,演示了如何使用`std::getline(std::cin, line)`函数读取用户输入的一行文本并输出:
```cpp
#include <iostream>
#include <string>
int main() {
std::string line;
std::getline(std::cin, line);
std::cout << line << std::endl;
return 0;
}
```
这段代码首先声明了一个字符串变量`line`,然后使用`std::getline(std::cin, line)`函数从标准输入流中读取一行文本,并将其存储在`line`变量中。最后,使用`std::cout`将读取到的文本输出到标准输出流。
相关推荐
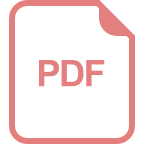
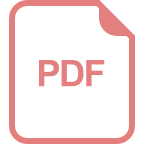












