用Python中的turtle 绘图,获取实时的年、月、日、时、分、秒的七段数码管
时间: 2023-05-31 19:01:47 浏览: 44
以下是一个示例代码,可以在Python中使用turtle库实时绘制年、月、日、时、分、秒的七段数码管:
```python
import turtle
import time
# 七段数码管的绘制函数
def draw_segment(digit, size):
if digit in [2, 3, 4, 5, 6, 8, 9]:
turtle.forward(size)
else:
turtle.penup()
turtle.forward(size)
turtle.pendown()
turtle.right(90)
if digit in [0, 1, 3, 4, 5, 6, 7, 8, 9]:
turtle.forward(size)
else:
turtle.penup()
turtle.forward(size)
turtle.pendown()
turtle.right(90)
if digit in [0, 2, 3, 5, 6, 8, 9]:
turtle.forward(size)
else:
turtle.penup()
turtle.forward(size)
turtle.pendown()
turtle.right(90)
if digit in [0, 2, 6, 8]:
turtle.forward(size)
else:
turtle.penup()
turtle.forward(size)
turtle.pendown()
turtle.right(90)
if digit in [0, 4, 5, 6, 8, 9]:
turtle.forward(size)
else:
turtle.penup()
turtle.forward(size)
turtle.pendown()
turtle.right(90)
if digit in [0, 2, 3, 5, 6, 7, 8, 9]:
turtle.forward(size)
else:
turtle.penup()
turtle.forward(size)
turtle.pendown()
turtle.right(90)
if digit in [0, 1, 2, 3, 4, 7, 8, 9]:
turtle.forward(size)
else:
turtle.penup()
turtle.forward(size)
turtle.pendown()
turtle.right(90)
turtle.penup()
turtle.forward(size*2)
turtle.right(180)
turtle.pendown()
# 获取当前时间
current_time = time.localtime()
# 设置turtle绘图窗口的大小和位置
screen = turtle.Screen()
screen.setup(800, 400)
screen.bgcolor("black")
screen.title("Seven Segment Display")
# 创建turtle对象
t = turtle.Turtle()
t.speed(0)
t.pensize(3)
t.penup()
t.goto(-300, 0)
t.pendown()
# 绘制年的七段数码管
t.color("red")
draw_segment(int(current_time.tm_year/1000), 20)
draw_segment(int(current_time.tm_year/100%10), 20)
draw_segment(int(current_time.tm_year/10%10), 20)
draw_segment(int(current_time.tm_year%10), 20)
# 绘制月的七段数码管
t.penup()
t.goto(-150, 0)
t.pendown()
t.color("green")
draw_segment(int(current_time.tm_mon/10), 20)
draw_segment(int(current_time.tm_mon%10), 20)
# 绘制日的七段数码管
t.penup()
t.goto(0, 0)
t.pendown()
t.color("blue")
draw_segment(int(current_time.tm_mday/10), 20)
draw_segment(int(current_time.tm_mday%10), 20)
# 绘制时的七段数码管
t.penup()
t.goto(150, 0)
t.pendown()
t.color("yellow")
draw_segment(int(current_time.tm_hour/10), 20)
draw_segment(int(current_time.tm_hour%10), 20)
# 绘制分的七段数码管
t.penup()
t.goto(300, 0)
t.pendown()
t.color("purple")
draw_segment(int(current_time.tm_min/10), 20)
draw_segment(int(current_time.tm_min%10), 20)
# 绘制秒的七段数码管
t.penup()
t.goto(450, 0)
t.pendown()
t.color("orange")
draw_segment(int(current_time.tm_sec/10), 20)
draw_segment(int(current_time.tm_sec%10), 20)
# 循环更新时间并重绘数码管
while True:
new_time = time.localtime()
if new_time.tm_sec != current_time.tm_sec:
current_time = new_time
t.clear()
t.penup()
t.goto(-300, 0)
t.pendown()
t.color("red")
draw_segment(int(current_time.tm_year/1000), 20)
draw_segment(int(current_time.tm_year/100%10), 20)
draw_segment(int(current_time.tm_year/10%10), 20)
draw_segment(int(current_time.tm_year%10), 20)
t.penup()
t.goto(-150, 0)
t.pendown()
t.color("green")
draw_segment(int(current_time.tm_mon/10), 20)
draw_segment(int(current_time.tm_mon%10), 20)
t.penup()
t.goto(0, 0)
t.pendown()
t.color("blue")
draw_segment(int(current_time.tm_mday/10), 20)
draw_segment(int(current_time.tm_mday%10), 20)
t.penup()
t.goto(150, 0)
t.pendown()
t.color("yellow")
draw_segment(int(current_time.tm_hour/10), 20)
draw_segment(int(current_time.tm_hour%10), 20)
t.penup()
t.goto(300, 0)
t.pendown()
t.color("purple")
draw_segment(int(current_time.tm_min/10), 20)
draw_segment(int(current_time.tm_min%10), 20)
t.penup()
t.goto(450, 0)
t.pendown()
t.color("orange")
draw_segment(int(current_time.tm_sec/10), 20)
draw_segment(int(current_time.tm_sec%10), 20)
time.sleep(0.1)
```
该代码使用turtle库绘制年、月、日、时、分、秒的七段数码管,同时使用time库实时获取当前时间并更新数码管的显示。运行该代码后,可以在窗口中看到实时的时间数码管显示。
相关推荐
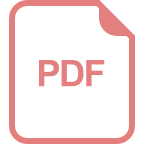
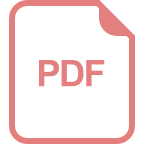












