设字符串采用单字符的链式存储结构,要求写一算法能实现删除串s从位置i开始长度为k的子串。 输入格式: 一共有两行,第一行有一串连续的字符串,并且以#结束。第二行i,k分别表示需要删除的位置(从1开始计数)和删除的长度(题目保证删除的长度不超过字符串的长度)。 输出格式: 输出删除后的字符串。 输入样例: It is never too late to mend.# 7 6 输出样例: It is too late to mend用c语言.
时间: 2024-03-27 17:37:34 浏览: 45
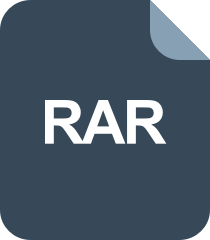
用串的链式存储实现插入,删除,查找,匹配等操作

以下是一个简单的 C 语言实现,使用了链表来存储字符串:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
char data; // 节点存储的字符
struct Node* next; // 指向下一个节点的指针
} Node;
// 定义链表结构体
typedef struct {
Node* head; // 指向链表头节点的指针
int length; // 链表长度
} LinkedList;
// 初始化链表
void initLinkedList(LinkedList* list) {
list->head = NULL;
list->length = 0;
}
// 在链表尾部插入节点
void insertNode(LinkedList* list, char data) {
Node* node = (Node*) malloc(sizeof(Node));
node->data = data;
node->next = NULL;
if (list->head == NULL) {
list->head = node;
} else {
Node* p = list->head;
while (p->next != NULL) {
p = p->next;
}
p->next = node;
}
list->length++;
}
// 删除从位置i开始长度为k的子串
void deleteSubstring(LinkedList* list, int i, int k) {
if (i < 1 || i > list->length || k < 1) {
return;
}
Node* p = list->head;
Node* prev = NULL;
int j = 1;
while (p != NULL && j < i) {
prev = p;
p = p->next;
j++;
}
if (p == NULL) {
return;
}
Node* q = p;
j = 0;
while (q != NULL && j < k) {
q = q->next;
j++;
}
if (prev == NULL) {
list->head = q;
} else {
prev->next = q;
}
list->length -= k;
while (p != q) {
Node* temp = p;
p = p->next;
free(temp);
}
}
// 输出链表
void printLinkedList(LinkedList* list) {
Node* p = list->head;
while (p != NULL) {
printf("%c", p->data);
p = p->next;
}
printf("\n");
}
int main() {
LinkedList list;
initLinkedList(&list);
char c;
scanf("%c", &c);
while (c != '#') {
insertNode(&list, c);
scanf("%c", &c);
}
int i, k;
scanf("%d %d", &i, &k);
deleteSubstring(&list, i, k);
printLinkedList(&list);
return 0;
}
```
注意在输入字符串时,最后一个字符是 `#`,读取时需要忽略。
阅读全文
相关推荐
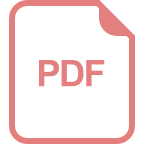


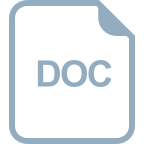












