java利用数组实现队列的基本功能:
时间: 2023-05-11 11:03:35 浏览: 116
Java利用数组实现队列的基本功能可以通过以下步骤实现:
1. 定义一个数组来存储队列元素,同时定义一个变量来记录队列的大小。
2. 定义两个指针front和rear,分别指向队列的头部和尾部。
3. 当队列为空时,front和rear指向同一个位置。
4. 当队列不为空时,front指向队列头部,rear指向队列尾部。
5. 入队操作时,将元素添加到rear指向的位置,并将rear指针向后移动一位。
6. 出队操作时,将front指向的元素弹出,并将front指针向后移动一位。
7. 队列满时,无法再进行入队操作。
下面是Java代码实现:
public class ArrayQueue {
private int[] queue;
private int front;
private int rear;
private int size;
public ArrayQueue(int capacity) {
queue = new int[capacity];
front = 0;
rear = 0;
size = 0;
}
public void enqueue(int element) {
if (size == queue.length) {
throw new IllegalStateException("Queue is full");
}
queue[rear] = element;
rear = (rear + 1) % queue.length;
size++;
}
public int dequeue() {
if (size == 0) {
throw new IllegalStateException("Queue is empty");
}
int element = queue[front];
front = (front + 1) % queue.length;
size--;
return element;
}
public boolean isEmpty() {
return size == 0;
}
public boolean isFull() {
return size == queue.length;
}
public int size() {
return size;
}
}
阅读全文
相关推荐






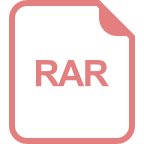







