#include <iostream> using namespace std; void delchar( char a[ ],char b ) { int i,j=0; for(i=0;a[i]!='\0';i++) if() { a[j]=a[i]; j++; } ; } int main() { char s[1024]; char b; cin.getline(s, 1024); cin >> b; delchar(s, b); cout << s; }优化代码
时间: 2024-03-18 17:40:42 浏览: 61
在 `delchar()` 函数中,需要添加判断条件来判断当前字符是否需要删除,同时可以优化循环语句。优化后的代码如下:
```c++
#include <iostream>
using namespace std;
void delchar(char a[], char b) {
int i = 0, j = 0;
while (a[i]) {
if (a[i] != b) {
a[j] = a[i];
j++;
}
i++;
}
a[j] = '\0';
}
int main() {
char s[1024];
char b;
cin.getline(s, 1024);
cin >> b;
delchar(s, b);
cout << s;
return 0;
}
```
其中,`while` 循环语句中的 `a[i]` 相当于 `a[i] != '\0'`,可以直接用来作为循环条件。在循环中,如果当前字符不等于需要删除的字符,则将当前字符赋值给 `a[j]`,并将 `j` 的值加 1。最后,将 `a[j]` 赋值为字符串结束符 `\0`,达到截断字符串的效果。
相关问题
#include <stdexcept> #include <string> #include "huffman_encoder.h" #include <chrono> // 添加计时器需要的头文件 #include <iostream> // 添加输出需要的头文件 using namespace std; using namespace std::chrono; // 添加计时器需要的命名空间 int main(int argc, char** argv) { assert(argc >= 1); string filename = argv[1]; zfish::HuffmanEncoder hec{ filename }; hec.run(); // 记录解压缩所花费的时间 auto start = high_resolution_clock::now(); hec.decode(); auto stop = high_resolution_clock::now(); auto duration = duration_cast<milliseconds>(stop - start); // 输出解压缩速度 cout << "解压缩速度:" << duration.count() << " 毫秒" << endl; getchar(); return 0; }decode的定义怎么定义,
根据代码中的上下文,可以猜测 `decode` 是 `HuffmanEncoder` 类的一个成员函数,用于解码压缩后的数据。以下是一个可能的 `decode` 函数的定义:
```c++
void HuffmanEncoder::decode() {
// implementation goes here
// 解码压缩后的数据
}
```
在这个例子中,`decode` 是 `HuffmanEncoder` 类的一个成员函数,没有返回值。具体实现根据压缩方式的不同而有所不同。
#include <iostream> using namespace std; class class0 { public: virtual ~class0(){} protected: char p; public: char getChar(); }; class class1 : public class0 { public: void printChar(); }; void class1::printChar() { cout << "True" << endl; } int main() { class1 c; c.printChar(); return 0; }
这段代码定义了两个类 `class0` 和 `class1`,其中 `class1` 继承了 `class0`。`class0` 定义了一个受保护的 char 类型的数据成员 `p` 和一个公有的成员函数 `getChar()`,`class1` 定义了一个公有的成员函数 `printChar()`。`class0` 的析构函数是虚函数。
在 `main()` 函数中,创建了一个 `class1` 类型的对象 `c`,然后调用了它的 `printChar()` 成员函数。该函数只是简单地将字符串 "True" 输出到标准输出流中,然后换行。最后返回 0。
需要注意的是,`class0` 的析构函数是虚函数,这意味着当 `class1` 类型的对象被销毁时,会自动调用 `class0` 的析构函数。由于 `class0` 的析构函数是空的,因此这个程序并不会有任何内存泄漏的问题。
阅读全文
相关推荐
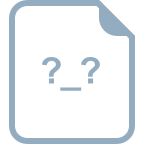
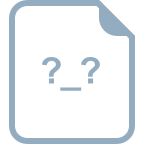







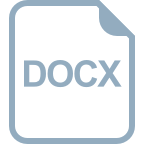
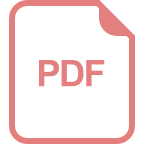





