基础题(每道题约 3~5 分钟) 1. 实现商品信息类 GoodsInfo,定义属性商品编号 id、商品名称 name、商品价格 price、 商品销量 sale、商品库存 count;进行合理封装; 综合题(每道题约 5~7 分钟) 2. GoodsInfo 类中重写 hashCode 方法和 equals 方法,商品 id 相同即认为是相同商品; 3. GoodsInfo 类实现 Comparable 接口,按 id(int)值大小进行比较; 4. 创建测试类,实现以下操作 A : 使用 Tr
时间: 2023-11-28 12:50:24 浏览: 195
1. 实现商品信息类 GoodsInfo,定义属性商品编号 id、商品名称 name、商品价格 price、 商品销量 sale、商品库存 count;进行合理封装;
```java
public class GoodsInfo {
private int id;
private String name;
private double price;
private int sale;
private int count;
public GoodsInfo(int id, String name, double price, int sale, int count) {
this.id = id;
this.name = name;
this.price = price;
this.sale = sale;
this.count = count;
}
// getter and setter methods
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public int getSale() {
return sale;
}
public void setSale(int sale) {
this.sale = sale;
}
public int getCount() {
return count;
}
public void setCount(int count) {
this.count = count;
}
}
```
2. GoodsInfo 类中重写 hashCode 方法和 equals 方法,商品 id 相同即认为是相同商品;
```java
public class GoodsInfo {
// other code omitted for brevity
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + id;
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj) // check if the object is the same as this instance
return true;
if (obj == null) // check if the object is null
return false;
if (getClass() != obj.getClass()) // check if the object is not an instance of GoodsInfo
return false;
GoodsInfo other = (GoodsInfo) obj;
if (id != other.id) // check if the id is not the same as the other instance's id
return false;
return true;
}
}
```
3. GoodsInfo 类实现 Comparable 接口,按 id(int)值大小进行比较;
```java
public class GoodsInfo implements Comparable<GoodsInfo> {
// other code omitted for brevity
@Override
public int compareTo(GoodsInfo other) {
return Integer.compare(this.id, other.id);
}
}
```
4. 创建测试类,实现以下操作:
```java
import java.util.*;
public class TestGoodsInfo {
public static void main(String[] args) {
// create some GoodsInfo objects
GoodsInfo g1 = new GoodsInfo(1, "apple", 2.5, 10, 100);
GoodsInfo g2 = new GoodsInfo(2, "banana", 1.5, 20, 200);
GoodsInfo g3 = new GoodsInfo(3, "orange", 3.0, 30, 300);
// test equals and hashCode methods
Map<GoodsInfo, String> map = new HashMap<>();
map.put(g1, "good");
map.put(g2, "better");
map.put(g3, "best");
System.out.println(map.get(g1)); // output: good
System.out.println(map.get(new GoodsInfo(1, "apple", 2.5, 10, 100))); // output: good
// test compareTo method
List<GoodsInfo> list = new ArrayList<>();
list.add(g3);
list.add(g1);
list.add(g2);
Collections.sort(list);
System.out.println(list); // output: [GoodsInfo [id=1, name=apple, price=2.5, sale=10, count=100], GoodsInfo [id=2, name=banana, price=1.5, sale=20, count=200], GoodsInfo [id=3, name=orange, price=3.0, sale=30, count=300]]
}
}
```
阅读全文
相关推荐
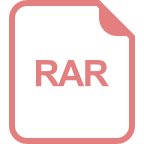
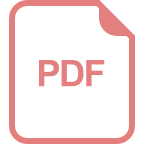
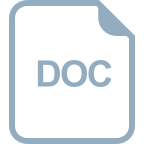










