用pytorch实现logistic回归,其中要有数据准备,线性方程,激活函数,损失函数,优化算法,模型可视化
时间: 2024-05-10 13:20:23 浏览: 127
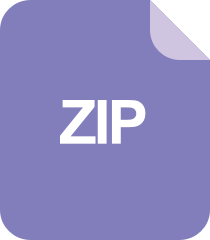
基于pytorch的线性回归模型,python
以下是一个简单的pytorch实现logistic回归的示例代码:
```
import torch
import torch.nn as nn
import numpy as np
import matplotlib.pyplot as plt
# 准备数据
np.random.seed(0)
X = np.random.randn(100, 2)
y = np.random.randint(0, 2, size=(100, 1))
X[y==0] += 2
# 转换为tensor
X = torch.from_numpy(X).float()
y = torch.from_numpy(y).float()
# 定义模型
class LogisticRegression(nn.Module):
def __init__(self, input_dim):
super(LogisticRegression, self).__init__()
self.linear = nn.Linear(input_dim, 1)
self.sigmoid = nn.Sigmoid()
def forward(self, x):
x = self.linear(x)
x = self.sigmoid(x)
return x
# 实例化模型
model = LogisticRegression(input_dim=2)
# 定义损失函数
criterion = nn.BCELoss()
# 定义优化器
optimizer = torch.optim.SGD(model.parameters(), lr=0.1)
# 训练模型
num_epochs = 1000
for epoch in range(num_epochs):
# 前向传播
y_pred = model(X)
loss = criterion(y_pred, y)
# 反向传播
optimizer.zero_grad()
loss.backward()
optimizer.step()
# 输出结果
if (epoch+1) % 100 == 0:
print('Epoch [{}/{}], Loss: {:.4f}'.format(epoch+1, num_epochs, loss.item()))
# 可视化结果
w, b = model.parameters()
w = w.detach().numpy()
b = b.detach().numpy()
plt.scatter(X[:, 0], X[:, 1], c=y.flatten())
x_min, x_max = X[:, 0].min() - 1, X[:, 0].max() + 1
y_min, y_max = X[:, 1].min() - 1, X[:, 1].max() + 1
xx, yy = np.meshgrid(np.arange(x_min, x_max, 0.1), np.arange(y_min, y_max, 0.1))
Z = 1 / (1 + np.exp(-(w[0]*xx + w[1]*yy + b)))
Z[Z >= 0.5] = 1
Z[Z < 0.5] = 0
plt.contourf(xx, yy, Z, alpha=0.2)
plt.show()
```
在此代码中,我们首先准备数据,然后定义了一个LogisticRegression类作为我们的模型。该类包含一个线性层和Sigmoid激活函数。我们使用BCELoss作为损失函数,SGD作为优化算法,进行1000次迭代训练。最后,我们将模型的参数可视化,以便更好地理解模型的决策边界。
阅读全文
相关推荐
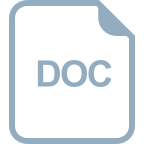
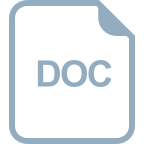


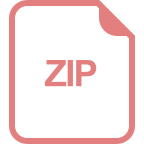
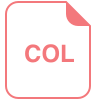
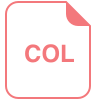
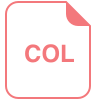
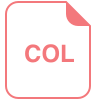
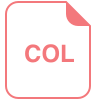
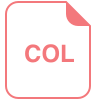
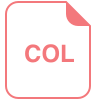
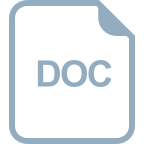
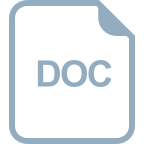