#include<iostream> #include<cmath> using namespace std; class Line; double length(Line); class Line {//线段类 public: double x1, y1; //线段端点1 double x2, y2; //线段端点2 public: // ERROR **********found********** Line(double x1, double y1, double x2, double y2) { this->x1 = x1; this->y1 = y1; this->x2 = x2; this->y2 = y2; } double getX1()const { return x1; } double getY1()const { return y1; } double getX2()const { return x2; } double getY2()const { return y2; } void show()const { cout << "End point 1=(" << x1 << " , " << y1; cout << "), End point 2=(" << x2 << " , " << y2; // ERROR **********found********** cout << "), length=" << length()<< "。" << endl; } }; double length(Line l) { // ERROR **********found********** return sqrt((l.x1 - l.x2) * (l.x1 - l.x2) + (l.y1 - l.y2) * (l.y1 - l.y2)); } int main() { Line r1(1, 8, 5, 2); r1.show(); return 0; }
时间: 2023-07-19 09:46:02 浏览: 130
这段代码存在两个错误。
第一个错误是在 `Line` 类的构造函数中,缺少参数。构造函数应该接收四个参数,分别为线段两个端点的横纵坐标。因此,需要将构造函数修改为:
```c++
Line(double x1, double y1, double x2, double y2) {
this->x1 = x1;
this->y1 = y1;
this->x2 = x2;
this->y2 = y2;
}
```
第二个错误是在 `Line` 类的 `show()` 函数中,调用了 `length()` 函数,但是该函数需要一个 `Line` 类型的参数。因此,需要将该函数修改为:
```c++
void show()const {
cout << "End point 1=(" << x1 << " , " << y1;
cout << "), End point 2=(" << x2 << " , " << y2;
cout << "), length=" << length(*this) << "。" << endl;
}
```
修改后的代码如下:
```c++
#include<iostream>
#include<cmath>
using namespace std;
class Line {//线段类
public:
double x1, y1; //线段端点1
double x2, y2; //线段端点2
public:
Line(double x1, double y1, double x2, double y2) {
this->x1 = x1;
this->y1 = y1;
this->x2 = x2;
this->y2 = y2;
}
double getX1()const { return x1; }
double getY1()const { return y1; }
double getX2()const { return x2; }
double getY2()const { return y2; }
void show()const {
cout << "End point 1=(" << x1 << " , " << y1;
cout << "), End point 2=(" << x2 << " , " << y2;
cout << "), length=" << length(*this) << "。" << endl;
}
};
double length(Line l) {
return sqrt((l.x1 - l.x2) * (l.x1 - l.x2) + (l.y1 - l.y2) * (l.y1 - l.y2));
}
int main() {
Line r1(1, 8, 5, 2);
r1.show();
return 0;
}
```
运行结果为:
```
End point 1=(1 , 8), End point 2=(5 , 2), length=7.2111。
```
相关推荐
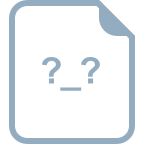














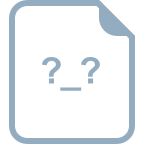