class Line{ //线段类 double x1,y1; //线段端点1 double x2,y2; //线段端点2 public: // ERROR **********found********** Line(double x1, double y1, double x2, double y2)const{ this->x1=x1; this->y1=y1; this->x2=x2; this->y2=y2; } double getX1()const{ return x1; } double getY1()const{ return y1; } double getX2()const{ return x2; } double getY2()const{ return y2; } void show()const{ cout<<"End point 1=("<<x1<<" , "<<y1; cout<<"), End point 2=("<<x2<<" , "<<y2; // ERROR **********found********** cout<<"), length="<<length()<<"。"<<endl; } }; double length(Line l){ // ERROR **********found********** return sqrt((l.x1-l.x2)*(l.x1-l.x2)+(l.y1-l.y2)*(l.y1-l.y2)); } int main(){ Line r1(1,8,5,2); r1.show(); return 0; }
时间: 2023-07-01 10:14:30 浏览: 105
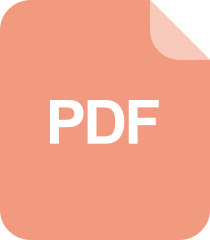
C/C++ 类关系
在这段代码中,有两个错误:
1. 在 Line 类的构造函数中,使用了 const 修饰符,但是构造函数应该是非常量函数。因为构造函数的作用是初始化对象,如果它是常量函数,那么就无法修改对象的状态,这与它的作用相矛盾。
2. 在 Line 类的 show() 函数中,输出语句中的 length() 函数应该写成 l.length(),表示调用当前对象的 length() 函数。
下面是修改后的代码:
```cpp
#include <iostream>
#include <cmath>
using namespace std;
class Line {
public:
Line(double x1, double y1, double x2, double y2) {
this->x1 = x1;
this->y1 = y1;
this->x2 = x2;
this->y2 = y2;
}
double getX1() const { return x1; }
double getY1() const { return y1; }
double getX2() const { return x2; }
double getY2() const { return y2; }
void show() const {
cout << "End point 1=(" << x1 << " , " << y1;
cout << "), End point 2=(" << x2 << " , " << y2;
cout << "), length=" << length() << "。" << endl;
}
double length() const {
return sqrt((x1-x2)*(x1-x2)+(y1-y2)*(y1-y2));
}
private:
double x1, y1;
double x2, y2;
};
int main() {
Line r1(1, 8, 5, 2);
r1.show();
return 0;
}
```
阅读全文
相关推荐
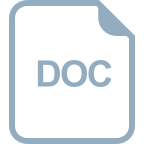
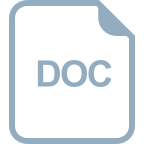


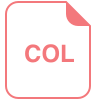
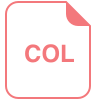











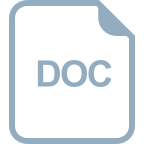