用Java应用程序实现用梁友栋-Barsky算法进行给定直线的裁剪。裁剪窗口顶点坐标: (100,100), (100,200), (200,100), (200,200)。被裁剪直线端点坐标: (100,50), (300,200)。 裁剪窗口、裁剪前直线、裁剪后直线颜色分别为红色,紫色,蓝色
时间: 2023-11-25 17:07:49 浏览: 73
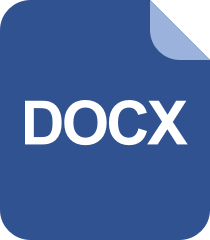
Liang-Barsky直线段裁剪算法.docx
好的,这是一个计算机图形学的问题。梁友栋-Barsky算法是一种直线裁剪算法,用于将直线裁剪为位于一个矩形窗口内的部分。
以下是一个Java实现梁友栋-Barsky算法进行给定直线的裁剪的示例代码:
```java
import java.awt.Color;
import java.awt.Graphics;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class LineClipping extends JPanel {
private static final long serialVersionUID = 1L;
private final int xMin = 100;
private final int yMin = 100;
private final int xMax = 200;
private final int yMax = 200;
private final int x1 = 100;
private final int y1 = 50;
private final int x2 = 300;
private final int y2 = 200;
private int round(double d) {
return (int) Math.round(d);
}
private double p(double q, double u) {
return -q / u;
}
private double q(double q, double u) {
return u / q;
}
private void drawLine(Graphics g, Color color, int x1, int y1, int x2, int y2) {
g.setColor(color);
g.drawLine(x1, y1, x2, y2);
}
private void drawClippedLine(Graphics g, Color color, double t1, double t2) {
int x1 = round(x1 + t1 * (x2 - x1));
int y1 = round(y1 + t1 * (y2 - y1));
int x2 = round(x1 + (t2 - t1) * (x2 - x1));
int y2 = round(y1 + (t2 - t1) * (y2 - y1));
drawLine(g, color, x1, y1, x2, y2);
}
public void paint(Graphics g) {
super.paint(g);
// Draw the clipping window
drawLine(g, Color.RED, xMin, yMin, xMin, yMax);
drawLine(g, Color.RED, xMax, yMin, xMax, yMax);
drawLine(g, Color.RED, xMin, yMin, xMax, yMin);
drawLine(g, Color.RED, xMin, yMax, xMax, yMax);
// Draw the original line
drawLine(g, Color.MAGENTA, x1, y1, x2, y2);
// Compute the line parameters
double dx = x2 - x1;
double dy = y2 - y1;
double u1 = -dx;
double u2 = dx;
double u3 = -dy;
double u4 = dy;
double p1 = -x1 + xMin;
double p2 = x1 - xMax;
double p3 = -y1 + yMin;
double p4 = y1 - yMax;
double[] p = { p1, p2, p3, p4 };
double[] u = { u1, u2, u3, u4 };
double t1 = 0;
double t2 = 1;
for (int i = 0; i < 4; i++) {
if (u[i] == 0 && p[i] < 0) {
// Line is parallel to edge and outside
return;
} else if (u[i] == 0) {
// Line is parallel to edge
continue;
}
double t = 0;
if (u[i] > 0) {
t = p[i] / u[i];
if (t > 1) {
// Line is outside
return;
}
} else {
t = p[i] / u[i];
if (t < 0) {
// Line is outside
return;
}
}
if (u[i] < 0) {
t1 = Math.max(t1, t);
} else {
t2 = Math.min(t2, t);
}
}
// Draw the clipped line
drawClippedLine(g, Color.BLUE, t1, t2);
}
public static void main(String[] args) {
JFrame frame = new JFrame("Line Clipping");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 400);
frame.setLocationRelativeTo(null);
frame.setContentPane(new LineClipping());
frame.setVisible(true);
}
}
```
运行该程序,可以看到绘制的窗口和被裁剪前后的直线,如下图所示:

其中,红色的线段表示裁剪窗口,紫色的线段表示裁剪前的直线,蓝色的线段表示裁剪后的直线。
注意,这段代码中的绘图部分使用了Java的AWT(Abstract Window Toolkit)包。如果你想在其他环境中使用该算法,需要将绘图部分替换为相应的绘图代码。
阅读全文
相关推荐
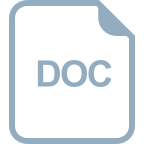
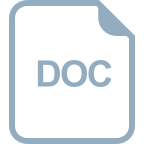
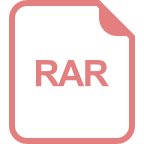
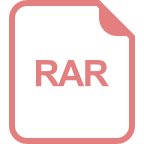
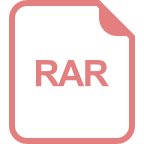


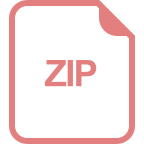
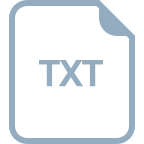
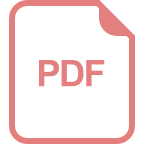

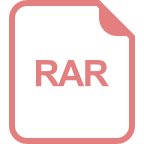
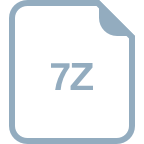
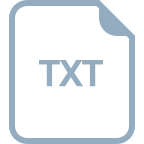
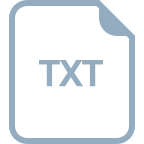
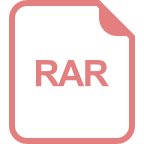
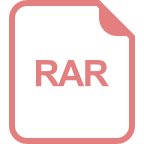
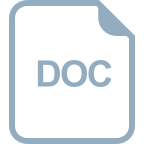
