java代码实现rsa加密
时间: 2024-06-10 10:07:32 浏览: 10
可以用以下的Java代码来实现RSA加密:
```java
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.PrivateKey;
import java.security.PublicKey;
import java.security.SecureRandom;
import javax.crypto.Cipher;
public class RSAEncryptor {
private static final String ALGORITHM = "RSA";
public static byte[] encrypt(byte[] data, PublicKey publicKey) throws Exception {
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
return cipher.doFinal(data);
}
public static byte[] decrypt(byte[] data, PrivateKey privateKey) throws Exception {
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.DECRYPT_MODE, privateKey);
return cipher.doFinal(data);
}
public static KeyPair generateKeyPair() throws Exception {
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance(ALGORITHM);
keyPairGenerator.initialize(2048, new SecureRandom());
return keyPairGenerator.generateKeyPair();
}
}
```
使用方法如下:
```java
// 生成密钥对
KeyPair keyPair = RSAEncryptor.generateKeyPair();
PrivateKey privateKey = keyPair.getPrivate();
PublicKey publicKey = keyPair.getPublic();
// 加密数据
String plaintext = "Hello, world!";
byte[] ciphertext = RSAEncryptor.encrypt(plaintext.getBytes(), publicKey);
// 解密数据
byte[] decryptedBytes = RSAEncryptor.decrypt(ciphertext, privateKey);
String decryptedPlaintext = new String(decryptedBytes);
System.out.println(decryptedPlaintext); // 输出:"Hello, world!"
```
这段代码实现了RSA加密和解密的功能。您可以使用`RSAEncryptor.generateKeyPair()`方法生成公钥和私钥,使用`RSAEncryptor.encrypt(...)`方法加密数据,使用`RSAEncryptor.decrypt(...)`方法解密数据。
相关推荐
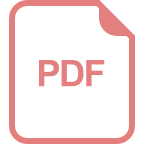
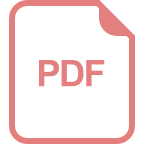
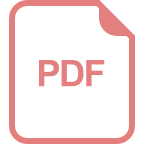














