matlab二分法求方程的所有根
时间: 2023-06-19 16:08:52 浏览: 232
二分法是一种寻找方程根的常用方法,具体步骤如下:
1. 确定方程根的区间 [a, b],使得 f(a) 和 f(b) 的符号不同。
2. 通过二分法将区间 [a, b] 分为两半,计算中点 c = (a+b)/2。
3. 计算 f(c) 的值,并判断它与 f(a) 和 f(b) 的符号是否相同。
4. 如果 f(c) 的符号与 f(a) 相同,则将区间缩小为 [c, b],否则将区间缩小为 [a, c]。
5. 重复步骤2-4,直到区间的长度小于指定的精度值。
6. 最终得到的 c 就是方程的一个根,如果要求所有根,则需要不断重复上述过程,直到找到所有根为止。
下面是一个用 MATLAB 实现二分法求方程根的示例代码:
```matlab
function [roots, iter] = bisection(f, a, b, tol, max_iter)
% Bisection method for finding roots of a function
% f: function handle
% a, b: initial interval
% tol: tolerance for stopping criteria
% max_iter: maximum number of iterations
% Initialize variables
fa = f(a);
fb = f(b);
iter = 0;
roots = [];
% Check if initial interval is valid
if (fa * fb) > 0
error('Initial interval is invalid!');
end
% Perform bisection
while abs(b-a) > tol && iter < max_iter
c = (a+b)/2;
fc = f(c);
if (fc * fa) < 0
b = c;
fb = fc;
else
a = c;
fa = fc;
end
iter = iter + 1;
% Check if c is a root
if abs(fc) <= tol
roots = [roots, c];
end
end
end
```
使用该函数可以求出方程的所有根,例如:
```matlab
% Define the function f(x) = x^3 - 6*x^2 + 11*x - 6
f = @(x) x^3 - 6*x^2 + 11*x - 6;
% Find all roots in the interval [0, 4]
[roots, iter] = bisection(f, 0, 4, 1e-6, 100);
% Display results
fprintf('Found %d roots in %d iterations:\n', length(roots), iter);
disp(roots);
```
阅读全文
相关推荐


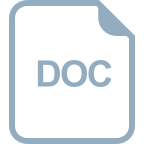










