class CWinBase { public: CWinBase(int x = 0, int y = 0, int w = 0, int h = 0); ~CWinBase(); void show(); // 显示界面 void win_run(); // 可以实现界面的操作:给编辑框能输入内容、按钮可以选择 virtual int doAction() = 0; // 按钮业务分发:不同的按钮处理不同的业务 protected: int x, y, w, h; vector<CtrlBase*> ctrlArry; // 统一管理某个界面的所有控件 int focusIndex; // 保存当前界面上选择的按钮在数组中的索引值 Database* db; // 数据库指针 void load_data_from_db(); // 从数据库中读取数据 }; void CWinBase::show() { load_data_from_db(); // 从数据库中读取数据并填充到控件中 // 显示界面 } void CWinBase::load_data_from_db() { // 连接数据库 db = new Database("localhost", "root", "password", "mydb"); db->connect(); // 读取数据 vector<Record> data = db->query("SELECT * FROM mytable"); // 填充控件 for (auto ctrl : ctrlArry) { if (ctrl->getType() == CtrlType::TEXTBOX) { // 如果是文本框,设置文本内容 dynamic_cast<TextBox*>(ctrl)->setText(data[0].getString("textbox_value")); } else if (ctrl->getType() == CtrlType::COMBOBOX) { // 如果是下拉框,添加选项 for (auto option : data[0].getStringList("combobox_options")) { dynamic_cast<ComboBox*>(ctrl)->addItem(option); } } else if (ctrl->getType() == CtrlType::LISTBOX) { // 如果是列表框,添加行数据 for (auto row : data) { dynamic_cast<ListBox*>(ctrl)->addRow(row.getString("listbox_value")); } } } // 断开数据库连接 db->disconnect(); delete db; }
时间: 2023-06-13 10:08:26 浏览: 68
这段代码定义了一个 CWinBase 类,该类表示一个窗口基类,包含窗口的位置、大小、控件列表和数据库指针等属性。该类还定义了一些方法,如 show() 方法用于将窗口显示出来,load_data_from_db() 方法用于从数据库中读取数据并填充到窗口控件中,win_run() 方法用于处理窗口的操作,doAction() 方法用于处理不同控件的业务分发。在 load_data_from_db() 方法中,通过调用数据库对象的方法连接数据库并查询数据,然后根据控件类型将查询结果填充到对应的控件中。最后,断开数据库连接并释放数据库对象。
相关问题
通过重写实现class CarWin :public CWinBase { public: CarWin(int x = 0, int y = 0, int w = 0, int h = 0); ~CarWin(); int doAction();//按钮分发:不同的按钮执行不同的业务 private: CLabel *title, *license_plate_lab, *total_lab, *remainder_lab; CEdit *license_plate_edit, *information_edit, *total_edit, *remainder_edit; CButt *query_btu, *storage_btu, *outbound_btu, *flushed_btu, *return_btu; };调用class CStorageWin :public CWinBase { public: CStorageWin(int x = 0, int y = 0, int w = 0, int h = 0); ~CStorageWin(); int doAction();//按钮分发:不同的按钮执行不同的业务 private: CLabel *title, *cpn_lab;//cpn=License plate number(车牌号) CEdit *cpn_edit; CButt *login_btu, *reg_btu; };里面的cpn_edit
可以通过以下方式来调用class CarWin中的cpn_edit:
1. 在CStorageWin中添加一个成员变量carWin,类型为CarWin*,并在构造函数中将它初始化为CarWin的实例。
2. 在CStorageWin的doAction方法中,通过carWin指针来访问CarWin的cpn_edit成员变量,例如carWin->cpn_edit。
示例代码如下:
class CStorageWin :public CWinBase {
public:
CStorageWin(int x = 0, int y = 0, int w = 0, int h = 0);
~CStorageWin();
int doAction();
private:
CLabel *title, *cpn_lab;
CEdit *cpn_edit;
CButt *login_btu, *reg_btu;
CarWin *carWin; // 添加一个CarWin指针
};
CStorageWin::CStorageWin(int x, int y, int w, int h) : CWinBase(x, y, w, h) {
title = new CLabel("车辆入库");
title->setPos(10, 10);
title->setSize(80, 20);
title->setParent(this);
cpn_lab = new CLabel("车牌号:");
cpn_lab->setPos(10, 40);
cpn_lab->setSize(80, 20);
cpn_lab->setParent(this);
cpn_edit = new CEdit();
cpn_edit->setPos(100, 40);
cpn_edit->setSize(120, 20);
cpn_edit->setParent(this);
login_btu = new CButt("入库");
login_btu->setPos(10, 80);
login_btu->setSize(60, 30);
login_btu->setParent(this);
reg_btu = new CButt("清空");
reg_btu->setPos(80, 80);
reg_btu->setSize(60, 30);
reg_btu->setParent(this);
carWin = new CarWin(); // 初始化CarWin指针
}
CStorageWin::~CStorageWin() {
delete title;
delete cpn_lab;
delete cpn_edit;
delete login_btu;
delete reg_btu;
delete carWin; // 释放CarWin指针
}
int CStorageWin::doAction() {
if (login_btu->isClick()) {
// 调用CarWin的doAction方法
carWin->doAction();
} else if (reg_btu->isClick()) {
cpn_edit->setText(""); // 清空车牌号输入框
}
return 0;
}
通过重写实现class CWinBase { public: CWinBase(int x = 0, int y = 0, int w = 0, int h = 0); ~CWinBase(); void show();//显示界面 void win_run();//可以实现界面的操作:给编辑框能输入内容、按钮可以选择 virtual int doAction()=0; //按钮业务分发:不同的按钮处理不同的业务 protected: int x, y, w, h; vector<CtrlBase*> ctrlArry;//统一管理某个界面的所有控件 int focusIndex; //保存当前界面上选择的按钮在数组中的索引值 };show()函数调用数据库数据
重写 CWinBase 类的 show() 函数,实现从数据库读取数据并显示在界面上的功能。可以按照以下步骤进行操作:
1. 在 CWinBase 类中添加一个指向数据库的指针成员变量 db,以及一个函数 load_data_from_db(),用于从数据库中读取数据。
2. 在 show() 函数中调用 load_data_from_db() 函数,将数据库中的数据读取出来,并填充到界面上的控件中。
3. 根据控件类型的不同,可以使用不同的方式来显示数据。例如,对于文本框,可以使用 setText() 函数来设置文本内容;对于下拉框,可以使用 addItem() 函数来添加选项;对于列表框,可以使用 addRow() 函数来添加行数据等。
下面是一个示例代码:
```
class CWinBase {
public:
CWinBase(int x = 0, int y = 0, int w = 0, int h = 0);
~CWinBase();
void show(); // 显示界面
void win_run(); // 可以实现界面的操作:给编辑框能输入内容、按钮可以选择
virtual int doAction() = 0; // 按钮业务分发:不同的按钮处理不同的业务
protected:
int x, y, w, h;
vector<CtrlBase*> ctrlArry; // 统一管理某个界面的所有控件
int focusIndex; // 保存当前界面上选择的按钮在数组中的索引值
Database* db; // 数据库指针
void load_data_from_db(); // 从数据库中读取数据
};
void CWinBase::show() {
load_data_from_db(); // 从数据库中读取数据并填充到控件中
// 显示界面
}
void CWinBase::load_data_from_db() {
// 连接数据库
db = new Database("localhost", "root", "password", "mydb");
db->connect();
// 读取数据
vector<Record> data = db->query("SELECT * FROM mytable");
// 填充控件
for (auto ctrl : ctrlArry) {
if (ctrl->getType() == CtrlType::TEXTBOX) {
// 如果是文本框,设置文本内容
dynamic_cast<TextBox*>(ctrl)->setText(data[0].getString("textbox_value"));
} else if (ctrl->getType() == CtrlType::COMBOBOX) {
// 如果是下拉框,添加选项
for (auto option : data[0].getStringList("combobox_options")) {
dynamic_cast<ComboBox*>(ctrl)->addItem(option);
}
} else if (ctrl->getType() == CtrlType::LISTBOX) {
// 如果是列表框,添加行数据
for (auto row : data) {
dynamic_cast<ListBox*>(ctrl)->addRow(row.getString("listbox_value"));
}
}
}
// 断开数据库连接
db->disconnect();
delete db;
}
```
相关推荐
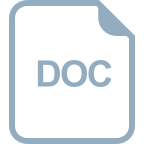
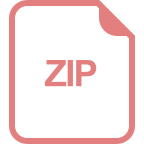
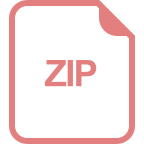













